Python请编写错题本代码
时间: 2024-01-21 13:17:00 浏览: 38
以下是一个简单的Python错题本代码示例:
```python
import os
class Question:
def __init__(self, question, answer):
self.question = question
self.answer = answer
def __str__(self):
return f"{self.question}: {self.answer}"
class Quiz:
def __init__(self, name):
self.name = name
self.questions = []
def add_question(self, question, answer):
self.questions.append(Question(question, answer))
def remove_question(self, index):
del self.questions[index]
def list_questions(self):
for i, question in enumerate(self.questions):
print(f"{i+1}. {question}")
def save_quiz(self):
with open(f"{self.name}.txt", "w") as f:
for question in self.questions:
f.write(f"{question.question}|{question.answer}\n")
def load_quiz(self):
if os.path.exists(f"{self.name}.txt"):
with open(f"{self.name}.txt", "r") as f:
for line in f:
question, answer = line.strip().split("|")
self.add_question(question, answer)
print(f"{self.name} quiz loaded.")
else:
print(f"{self.name} quiz does not exist.")
def take_quiz(self):
score = 0
total = len(self.questions)
for question in self.questions:
user_answer = input(f"{question.question}: ")
if user_answer == question.answer:
score += 1
print(f"Your score is {score}/{total}")
def clear_quiz(self):
self.questions = []
if os.path.exists(f"{self.name}.txt"):
os.remove(f"{self.name}.txt")
print(f"{self.name} quiz deleted.")
else:
print(f"{self.name} quiz does not exist.")
```
这个代码实现了一个`Question`类和一个`Quiz`类,可以用来创建错题本。你可以通过以下方式来使用这个代码:
```python
# 创建一个名为"python"的Quiz
python_quiz = Quiz("python")
# 添加问题和答案
python_quiz.add_question("What is the output of print(2+3*4)?", "14")
python_quiz.add_question("What is the keyword used for creating a function?", "def")
python_quiz.add_question("What is the output of print('hello'[::-1])?", "olleh")
# 列出所有问题和答案
python_quiz.list_questions()
# 保存Quiz
python_quiz.save_quiz()
# 加载Quiz
python_quiz.load_quiz()
# 进行Quiz
python_quiz.take_quiz()
# 清空Quiz
python_quiz.clear_quiz()
```
这个代码示例可以让你创建一个错题本,添加问题和答案,列出所有问题和答案,保存Quiz,加载Quiz,进行Quiz和清空Quiz。但是,请注意这个代码示例仅仅是一个简单的实现,如果你需要更复杂的功能,你需要根据自己的需要进行修改和扩展。
相关推荐
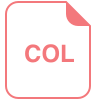
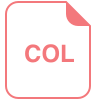
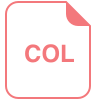
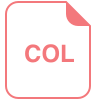
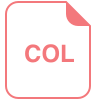









