使用typeid判读子类有没有重写父类的虚接口
时间: 2023-09-06 09:04:58 浏览: 101
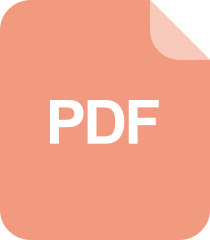
c++ typeid关键字的使用
### 回答1:
可以使用 typeid 来判断子类是否重写了父类的虚函数。
例如:
```
class Parent {
public:
virtual void func() {}
};
class Child : public Parent {
public:
void func() override {}
};
int main() {
Parent* p = new Child;
if (typeid(*p) == typeid(Child)) {
cout << "Child has overridden Parent's virtual function." << endl;
}
}
```
这里的代码会输出 "Child has overridden Parent's virtual function.",因为 Child 类重写了 Parent 类的 func() 虚函数。
注意,如果子类没有重写父类的虚函数,上面的判断会返回 false。
### 回答2:
使用typeid判断子类是否重写了父类的虚接口,可以通过以下方法实现。
首先,通过typeid操作符可以返回一个类型的标识信息,包括类型的名称和一些其他的信息。在子类中,可以通过比较typeid返回的类型信息是否相同来判断是否重写了父类的虚接口。
具体操作如下:
1. 首先,定义一个父类和一个子类,父类中含有一个虚接口。
```
class Parent {
public:
virtual void virtualFunc() {
cout << "父类的虚接口" << endl;
}
};
class Child : public Parent {
public:
void virtualFunc() {
cout << "子类重写了父类的虚接口" << endl;
}
};
```
2. 在程序中使用typeid判断子类是否重写了父类的虚接口。
```
Parent* parentPtr = new Parent();
Parent* childPtr = new Child();
if (typeid(*parentPtr) != typeid(*childPtr)) {
cout << "子类重写了父类的虚接口" << endl;
} else {
cout << "子类没有重写父类的虚接口" << endl;
}
```
在上述代码中,我们首先创建了一个`Parent`类和一个`Child`类的指针对象,然后通过typeid操作符判断这两个对象所对应的类型是否相同。如果不相同,则表示子类重写了父类的虚接口;如果相同,则表示子类没有重写父类的虚接口。
通过以上方法,我们可以使用typeid判断子类是否重写了父类的虚接口。
### 回答3:
使用 `typeid` 可以判断子类是否重写了父类的虚接口。首先,需要将父类的虚接口定义为纯虚函数(即在父类中设定接口的函数体为`= 0`),以确保父类只能有接口定义,而不能有实现。
接下来,在子类中通过重写父类的虚接口来实现具体的功能。如果子类重写了父类的虚接口,那么使用 `typeid` 判断子类对象时,其类型会被准确地识别为子类的类型,而不是父类的类型。
下面给出一个示例:
```cpp
#include <iostream>
#include <typeinfo>
using namespace std;
class Base {
public:
virtual void foo() = 0;
};
class Derived : public Base {
public:
void foo() override {
cout << "This is the derived class." << endl;
}
};
int main() {
Base* basePtr = new Derived();
if(typeid(*basePtr) == typeid(Derived)) {
cout << "The derived class has overwritten the virtual interface of the base class." << endl;
}
else {
cout << "The derived class has not overwritten the virtual interface of the base class." << endl;
}
delete basePtr;
return 0;
}
```
在上述示例中,我们定义了一个父类 `Base`,其中有一个纯虚函数 `foo`,然后定义了一个子类 `Derived`,并且在子类中重写了父类的虚接口 `foo`。
在 `main` 函数中,我们创建了一个 `Derived` 类的对象指针,并将其赋给了一个 `Base` 类型的指针。然后通过 `typeid` 将指针指向的对象类型与 `Derived` 类型进行比较。由于 `Derived` 类重写了 `Base` 类的虚接口,所以比较结果为真,表明子类重写了父类的虚接口。
【注意】需要包含 `typeinfo` 头文件并使用 `typeid`,以及使用虚函数和纯虚函数来实现多态性。
阅读全文
相关推荐
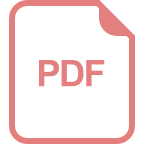
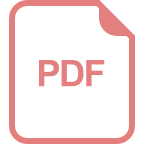


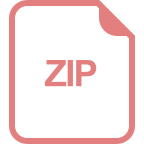












