用java写一个从键盘读取产品信息,并创建类product的实例。产品信息应该由三个值组成,都输入在同一行上,并用下划线()分隔。第一个值应该是一个表示产品名称的非空字符串。第二个值应该是一个正整数,表示产品的数量。第三个值应该是一个正的双精度数,表示价格。使用java.util.StringTokenizer提取三个va验证用户的输入: 如果用户输入的值超过3个,则返回错误提示。 如果用户输入的值少于3个,则返回错误提示。 如果用户输入的数量不是有效整数,则捕获并输出java.lang.NumberFormatException。 如果用户输入的价格不是有效的双精度数,则捕获并输出java.lang.NumberFormatException。 如果用户输入的数量为负数或零,则显示错误消息。 如果用户输入的数量为负数或零,则显示错误消息。 您的实现显示的错误消息应该与示例可执行文件显示的错误消息相匹配。 如果输入无效,readProduct将重新提示用户输入新的输入。否则,它将使用指定的名称、数量和价格创建一个新的Product对象,并将对新对象的引用返回给调用方法。
时间: 2023-05-19 11:01:45 浏览: 91
以下是用Java编写的从键盘读取产品信息并创建Product类实例的代码:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Product product = readProduct();
System.out.println("Product: " + product.getName() + ", Quantity: " + product.getQuantity() + ", Price: " + product.getPrice());
}
public static Product readProduct() {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Enter product information (name_quantity_price): ");
String input = scanner.nextLine();
String[] tokens = input.split("_");
if (tokens.length != 3) {
System.out.println("Error: Invalid input. Please enter name_quantity_price.");
continue;
}
String name = tokens[0];
int quantity;
double price;
try {
quantity = Integer.parseInt(tokens[1]);
price = Double.parseDouble(tokens[2]);
} catch (NumberFormatException e) {
System.out.println("Error: Invalid input. Quantity and price must be valid integers and doubles, respectively.");
continue;
}
if (quantity <= 0) {
System.out.println("Error: Quantity must be a positive integer.");
continue;
}
if (price <= 0) {
System.out.println("Error: Price must be a positive double.");
continue;
}
return new Product(name, quantity, price);
}
}
}
class Product {
private String name;
private int quantity;
private double price;
public Product(String name, int quantity, double price) {
this.name = name;
this.quantity = quantity;
this.price = price;
}
public String getName() {
return name;
}
public int getQuantity() {
return quantity;
}
public double getPrice() {
return price;
}
}
```
当用户输入无效时,程序会显示相应的错误消息并要求用户重新输入。如果输入有效,则程序将创建一个新的Product对象并返回对该对象的引用。
阅读全文
相关推荐
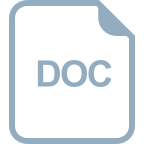
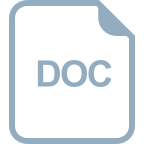
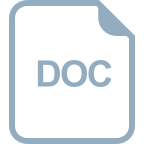

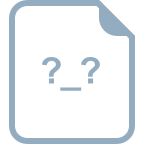
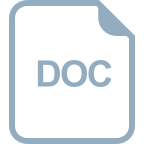
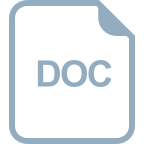
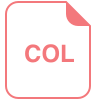
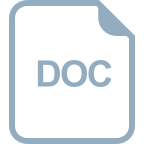
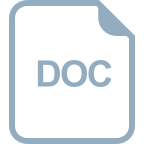
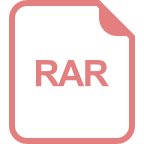
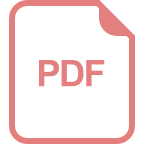
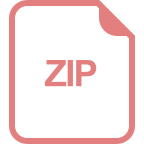
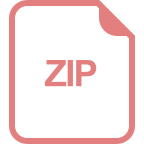
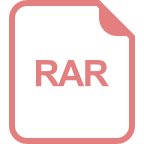
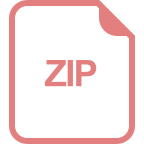