线性探测法c语言头文件,开放定址散列表(线性探测法)实现文件C语言
时间: 2024-03-09 22:47:30 浏览: 69
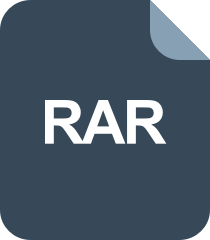
C语言头文件 LIST C语言头文件 LIST

以下是一个简单的开放定址散列表(线性探测法)的实现代码,包括头文件和实现文件:
头文件 hashtable.h:
```c
#ifndef HASHTABLE_H
#define HASHTABLE_H
#define TABLE_SIZE 1000
typedef struct {
int key;
int value;
} HashNode;
typedef struct {
HashNode *nodes;
int size;
} HashTable;
HashTable* createHashTable();
void destroyHashTable(HashTable *table);
void insert(HashTable *table, int key, int value);
int get(HashTable *table, int key);
#endif
```
实现文件 hashtable.c:
```c
#include <stdlib.h>
#include "hashtable.h"
HashTable* createHashTable() {
HashTable *table = (HashTable*) malloc(sizeof(HashTable));
table->nodes = (HashNode*) calloc(TABLE_SIZE, sizeof(HashNode));
table->size = TABLE_SIZE;
return table;
}
void destroyHashTable(HashTable *table) {
free(table->nodes);
free(table);
}
void insert(HashTable *table, int key, int value) {
int hash = key % table->size;
while (table->nodes[hash].value != 0) {
hash = (hash + 1) % table->size;
}
table->nodes[hash].key = key;
table->nodes[hash].value = value;
}
int get(HashTable *table, int key) {
int hash = key % table->size;
while (table->nodes[hash].value != 0) {
if (table->nodes[hash].key == key) {
return table->nodes[hash].value;
}
hash = (hash + 1) % table->size;
}
return 0;
}
```
在主程序中,可以使用以下方法来使用这个散列表:
```c
#include <stdio.h>
#include "hashtable.h"
int main() {
HashTable *table = createHashTable();
insert(table, 1, 100);
insert(table, 2, 200);
insert(table, 3, 300);
printf("%d\n", get(table, 2));
destroyHashTable(table);
return 0;
}
```
阅读全文
相关推荐
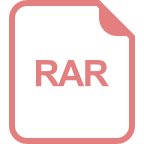
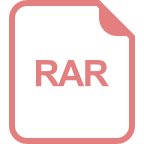
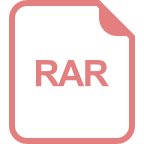
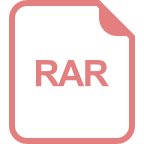
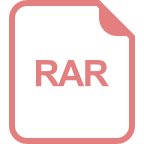
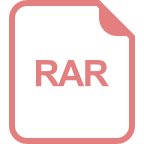
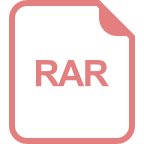
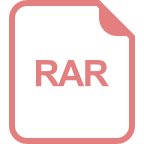
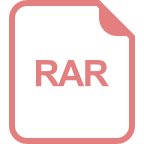
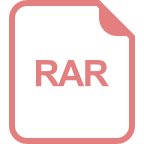
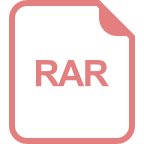
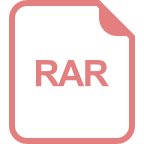
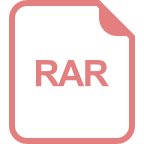
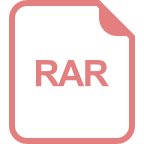
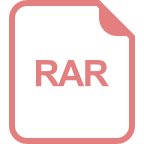
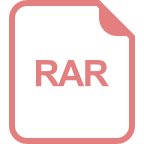
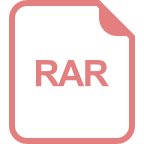