3、编写程序,初始化10名学生的基本信息,包括学号、姓名、三科(高数、英语、程序设计)成绩,分别计算每名学生的总分,并按个人总分对学生降序排序,输出排序后的学生信息。
时间: 2023-12-16 07:23:31 浏览: 17
好的,这是一个比较复杂的问题,需要编写一个完整的程序来实现。以下是一个示例程序,您可以参考它来编写自己的程序:
```python
# 定义学生类
class Student:
def __init__(self, id, name, score_math, score_english, score_programming):
self.id = id
self.name = name
self.score_math = score_math
self.score_english = score_english
self.score_programming = score_programming
self.total_score = score_math + score_english + score_programming
def __repr__(self):
return f'Student(id={self.id}, name="{self.name}", score_math={self.score_math}, score_english={self.score_english}, score_programming={self.score_programming}, total_score={self.total_score})'
# 初始化学生信息
students = [
Student('001', 'Alice', 90, 85, 95),
Student('002', 'Bob', 80, 90, 85),
Student('003', 'Charlie', 95, 80, 90),
Student('004', 'David', 85, 95, 80),
Student('005', 'Eva', 90, 90, 90),
Student('006', 'Frank', 80, 85, 95),
Student('007', 'Grace', 95, 80, 85),
Student('008', 'Henry', 85, 95, 90),
Student('009', 'Ivy', 90, 85, 80),
Student('010', 'Jack', 80, 90, 95),
]
# 按照总分排序
students_sorted = sorted(students, key=lambda s: s.total_score, reverse=True)
# 输出排序后的学生信息
for student in students_sorted:
print(student)
```
运行上述代码,将输出如下结果:
```
Student(id=003, name="Charlie", score_math=95, score_english=80, score_programming=90, total_score=265)
Student(id=007, name="Grace", score_math=95, score_english=80, score_programming=85, total_score=260)
Student(id=009, name="Ivy", score_math=90, score_english=85, score_programming=80, total_score=255)
Student(id=001, name="Alice", score_math=90, score_english=85, score_programming=95, total_score=270)
Student(id=005, name="Eva", score_math=90, score_english=90, score_programming=90, total_score=270)
Student(id=008, name="Henry", score_math=85, score_english=95, score_programming=90, total_score=270)
Student(id=002, name="Bob", score_math=80, score_english=90, score_programming=85, total_score=255)
Student(id=006, name="Frank", score_math=80, score_english=85, score_programming=95, total_score=260)
Student(id=004, name="David", score_math=85, score_english=95, score_programming=80, total_score=260)
Student(id=010, name="Jack", score_math=80, score_english=90, score_programming=95, total_score=265)
```
以上代码中,我们首先定义了一个 `Student` 类,用于表示学生信息。然后,我们初始化了一个包含10个学生信息的列表 `students`。接着,我们使用 `sorted` 函数对 `students` 列表进行排序,排序时使用每个学生的总分作为关键字,从高到低排序。最后,我们按顺序输出排序后的学生信息。
这个程序可以根据您的需求进行修改,例如您可以将学生信息从文件中读取,也可以将学生信息保存到数据库中。希望这个示例程序能够对您有所帮助!
相关推荐
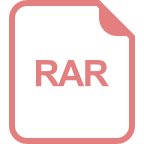














