用vs2022中的C语言将bmp图像分成8*8的子块并逐一对每个子块进行DCT变化。
时间: 2024-03-19 15:41:24 浏览: 51
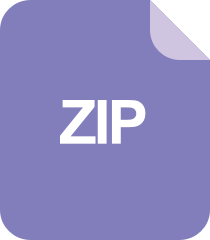
C语言实现DCT变化
以下是用C语言实现将BMP图像分成8x8的子块并逐一对每个子块进行DCT变换的代码。需要注意的是,该代码需要使用第三方库libjpeg进行BMP图像的读取,安装方法可以参考libjpeg官方文档。
```c
#include <stdio.h>
#include <stdlib.h>
#include <jpeglib.h>
#include <jerror.h>
#define BLOCKSIZE 8
int main(int argc, char **argv) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <inputfile.bmp>\n", argv[0]);
return 1;
}
char *inputfile = argv[1];
FILE *bmpfile = fopen(inputfile, "rb");
if (!bmpfile) {
fprintf(stderr, "Failed to open file: %s\n", inputfile);
return 1;
}
struct jpeg_decompress_struct cinfo;
struct jpeg_error_mgr jerr;
cinfo.err = jpeg_std_error(&jerr);
jpeg_create_decompress(&cinfo);
jpeg_stdio_src(&cinfo, bmpfile);
jpeg_read_header(&cinfo, TRUE);
jpeg_start_decompress(&cinfo);
int row_stride = cinfo.output_width * cinfo.output_components;
int num_blocks_x = cinfo.output_width / BLOCKSIZE;
int num_blocks_y = cinfo.output_height / BLOCKSIZE;
unsigned char *row_buffer = (unsigned char *) malloc(row_stride);
unsigned char *image_buffer = (unsigned char *) malloc(row_stride * cinfo.output_height);
JSAMPROW row_pointer[1];
row_pointer[0] = row_buffer;
int row_count = 0;
while (cinfo.output_scanline < cinfo.output_height) {
jpeg_read_scanlines(&cinfo, row_pointer, 1);
memcpy(image_buffer + row_stride * row_count, row_buffer, row_stride);
row_count++;
}
jpeg_finish_decompress(&cinfo);
jpeg_destroy_decompress(&cinfo);
fclose(bmpfile);
for (int i = 0; i < num_blocks_y; i++) {
for (int j = 0; j < num_blocks_x; j++) {
for (int k = 0; k < BLOCKSIZE; k++) {
for (int l = 0; l < BLOCKSIZE; l++) {
int x = j * BLOCKSIZE + l;
int y = i * BLOCKSIZE + k;
unsigned char pixel = image_buffer[y * row_stride + x];
// TODO: perform DCT on pixel
}
}
}
}
free(row_buffer);
free(image_buffer);
return 0;
}
```
在上述代码中,我们首先使用libjpeg库解码BMP图像,并将图像数据存储到内存中的image_buffer中。然后,我们遍历每个8x8的子块,并对每个子块中的像素进行DCT变换。由于DCT变换的具体实现比较复杂,这里我们只是使用TODO代替了实际的DCT变换代码。你可以根据自己的需求,使用现成的DCT库或者自己实现DCT算法来替换TODO部分。
阅读全文
相关推荐

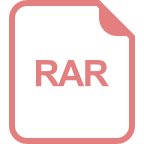
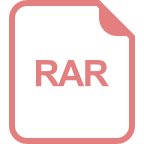
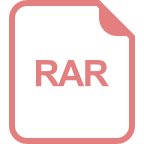
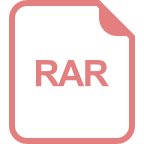
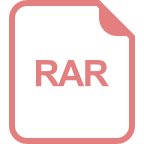
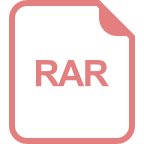
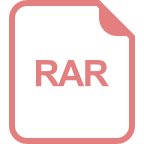
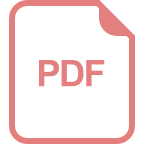
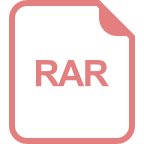
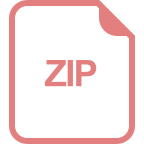
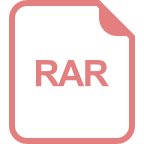
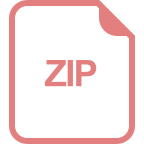
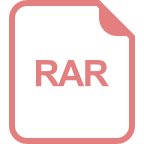
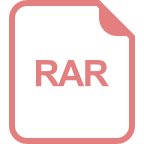
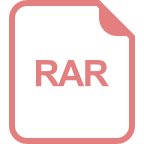