在Visual Studio 2022中用C语言中编写将图像分成8×8的子块,并逐一对每个子块进行DCT变换,并对变换后的数据进行保存
时间: 2024-03-26 10:34:56 浏览: 22
以下是一个使用 C 语言在 Visual Studio 2022 中实现将图像分成 8x8 的子块,并逐一对每个子块进行 DCT 变换,并对变换后的数据进行保存的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define WIDTH 640
#define HEIGHT 480
#define BLOCK_SIZE 8
unsigned char image[WIDTH * HEIGHT];
double alpha(int u) {
if (u == 0) {
return 1.0 / sqrt(2.0);
} else {
return 1.0;
}
}
void dct(double *block, double *dct_block) {
int u, v, i, j;
double cu, cv, sum;
for (u = 0; u < BLOCK_SIZE; u++) {
for (v = 0; v < BLOCK_SIZE; v++) {
sum = 0.0;
cu = alpha(u);
cv = alpha(v);
for (i = 0; i < BLOCK_SIZE; i++) {
for (j = 0; j < BLOCK_SIZE; j++) {
sum += block[i * BLOCK_SIZE + j] * cos((2 * i + 1) * u * M_PI / (2 * BLOCK_SIZE)) * cos((2 * j + 1) * v * M_PI / (2 * BLOCK_SIZE));
}
}
dct_block[u * BLOCK_SIZE + v] = cu * cv * sum / sqrt(2 * BLOCK_SIZE);
}
}
}
void process_block(unsigned char *image, int width, int height, int x, int y, double *dct_block) {
int i, j;
double block[BLOCK_SIZE * BLOCK_SIZE];
// 构造 8x8 的子块
for (i = 0; i < BLOCK_SIZE; i++) {
for (j = 0; j < BLOCK_SIZE; j++) {
block[i * BLOCK_SIZE + j] = (double) image[(y + i) * width + (x + j)];
}
}
// 执行 DCT 变换
dct(block, dct_block);
}
int main() {
FILE *fp_in, *fp_out;
char *filename_in = "input.raw";
char *filename_out = "output.raw";
double dct_block[BLOCK_SIZE * BLOCK_SIZE];
int n_blocks_wide = WIDTH / BLOCK_SIZE;
int n_blocks_high = HEIGHT / BLOCK_SIZE;
int x, y, i, j;
// 读取输入文件
if ((fp_in = fopen(filename_in, "rb")) == NULL) {
fprintf(stderr, "Error opening file %s\n", filename_in);
exit(1);
}
fread(image, sizeof(unsigned char), WIDTH * HEIGHT, fp_in);
fclose(fp_in);
// 对每个 8x8 的子块执行 DCT 变换,并保存结果
if ((fp_out = fopen(filename_out, "wb")) == NULL) {
fprintf(stderr, "Error opening file %s\n", filename_out);
exit(1);
}
for (y = 0; y < HEIGHT; y += BLOCK_SIZE) {
for (x = 0; x < WIDTH; x += BLOCK_SIZE) {
process_block(image, WIDTH, HEIGHT, x, y, dct_block);
fwrite(dct_block, sizeof(double), BLOCK_SIZE * BLOCK_SIZE, fp_out);
}
}
fclose(fp_out);
return 0;
}
```
在上面的代码中,我们首先定义了一个图像数组 `image`,以及一个常量 `BLOCK_SIZE`,表示每个子块的大小为 8x8。我们还定义了一个 `alpha` 函数,用于计算 DCT 变换中的 alpha 值。然后,我们定义了一个 `dct` 函数,它执行 DCT 变换。该函数将每个子块的值乘以相应的 cos 值,并将结果保存到 `dct_block` 数组中。最后,我们定义了一个 `process_block` 函数,它构造 8x8 的子块,并对其执行 DCT 变换。
在 `main` 函数中,我们首先读取输入文件,并计算图像中有多少个 8x8 的子块。然后,我们对每个子块执行 `process_block` 函数,将结果保存到输出文件中。注意,输出文件中保存的是 `dct_block` 数组的内容,即经过 DCT 变换后的数据,而不是原始的子块数据。
相关推荐
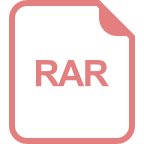














