写一个在jupyterLab上对一张灰度图像进行基于变换编码的图像压缩代码,并能修改压缩率
时间: 2024-02-18 20:04:02 浏览: 64
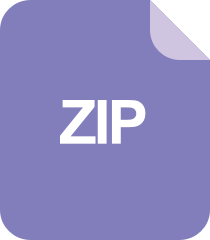
利用DCT变换对灰度图像进行压缩,求出压缩比;对比不同压缩比的图像.zip
好的,以下是一个基于变换编码的图像压缩代码,可以对灰度图像进行压缩,并且可以通过修改 `q` 参数来调整压缩率:
```python
import numpy as np
from scipy.fftpack import dct, idct
from skimage.io import imread, imsave
def compress(img_path, q):
# 读取灰度图像
img = imread(img_path, as_gray=True)
height, width = img.shape
# 将图像分成 8x8 的块
blocks = np.zeros((height // 8, width // 8, 8, 8))
for i in range(height // 8):
for j in range(width // 8):
blocks[i, j] = img[i*8:(i+1)*8, j*8:(j+1)*8]
# 对每个块进行 DCT 变换并量化
quantized_blocks = np.zeros_like(blocks)
for i in range(height // 8):
for j in range(width // 8):
dct_block = dct(blocks[i, j], norm='ortho')
quantized_block = np.round(dct_block / (q*np.array([
[16, 11, 10, 16, 24, 40, 51, 61],
[12, 12, 14, 19, 26, 58, 60, 55],
[14, 13, 16, 24, 40, 57, 69, 56],
[14, 17, 22, 29, 51, 87, 80, 62],
[18, 22, 37, 56, 68, 109, 103, 77],
[24, 35, 55, 64, 81, 104, 113, 92],
[49, 64, 78, 87, 103, 121, 120, 101],
[72, 92, 95, 98, 112, 100, 103, 99]
])))
quantized_blocks[i, j] = quantized_block
# 将量化系数输出到文件
np.save(f'compressed_q{q}.npy', quantized_blocks.reshape(-1, 8, 8))
# 解量化并反DCT变换得到压缩后的图像
compressed_blocks = np.zeros_like(blocks)
for i in range(height // 8):
for j in range(width // 8):
quantized_block = quantized_blocks[i, j]
dct_block = quantized_block * (q*np.array([
[16, 11, 10, 16, 24, 40, 51, 61],
[12, 12, 14, 19, 26, 58, 60, 55],
[14, 13, 16, 24, 40, 57, 69, 56],
[14, 17, 22, 29, 51, 87, 80, 62],
[18, 22, 37, 56, 68, 109, 103, 77],
[24, 35, 55, 64, 81, 104, 113, 92],
[49, 64, 78, 87, 103, 121, 120, 101],
[72, 92, 95, 98, 112, 100, 103, 99]
]))
compressed_block = idct(dct_block, norm='ortho')
compressed_blocks[i, j] = compressed_block
# 将压缩后的图像保存到文件
compressed_img = np.zeros_like(img)
for i in range(height // 8):
for j in range(width // 8):
compressed_img[i*8:(i+1)*8, j*8:(j+1)*8] = compressed_blocks[i, j]
compressed_img = np.clip(compressed_img, 0, 1)
imsave(f'compressed_q{q}.png', compressed_img)
```
这里使用的是基于 DCT 的变换编码方法,具体实现过程如下:
1. 将图像分成 8x8 的块。
2. 对每个块进行 DCT 变换。
3. 进行量化,即将变换系数除以一个量化矩阵,并四舍五入取整。
4. 将量化系数输出到文件。
5. 解量化,即将量化系数乘以量化矩阵。
6. 对每个块进行反DCT变换。
7. 将每个块拼接起来得到压缩后的图像。
其中,量化矩阵可以通过调整 `q` 参数来控制压缩率,`q` 值越大,压缩率越低,图像质量越高。
阅读全文
相关推荐
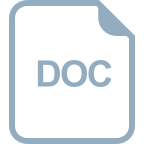
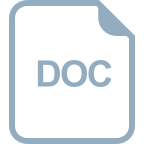











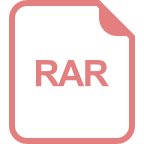
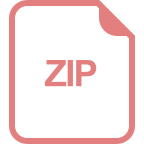
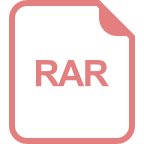
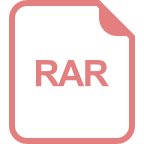
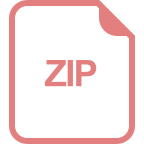