1.单链表的基本操作及应用 ①创建 ②插入 ③删除 ④查找 ⑤显示 通讯录设计(应用)
时间: 2024-01-01 18:06:55 浏览: 87
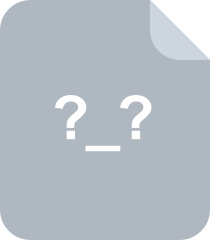
单链表的创建、插入、删除等基本操作

好的,以下是单链表的基本操作及应用的实现思路:
1. 创建单链表
创建单链表需要定义一个链表节点结构体,包含数据域和指针域。然后通过循环创建节点并将它们链接起来,最后返回链表的头指针。
```
struct Node {
int data; // 数据域
Node* next; // 指针域
};
Node* createList() {
Node* head = new Node; // 头节点不存储数据
head->next = nullptr;
Node* tail = head; // 尾指针,初始指向头节点
int n; // 节点个数
cin >> n;
for (int i = 0; i < n; i++) {
Node* node = new Node;
cin >> node->data;
node->next = nullptr;
tail->next = node;
tail = node;
}
return head;
}
```
2. 插入节点
插入节点分为头插法和尾插法。头插法将新节点插入到链表头部,尾插法将新节点插入到链表尾部。
```
// 头插法
void insertHead(Node* head, int value) {
Node* node = new Node;
node->data = value;
node->next = head->next;
head->next = node;
}
// 尾插法
void insertTail(Node* head, int value) {
Node* tail = head;
while (tail->next != nullptr) {
tail = tail->next;
}
Node* node = new Node;
node->data = value;
node->next = nullptr;
tail->next = node;
}
```
3. 删除节点
删除节点需要先找到待删除节点的前一个节点,然后将前一个节点的指针域指向待删除节点的后一个节点,最后释放待删除节点的内存空间。
```
void deleteNode(Node* head, int value) {
Node* p = head;
while (p->next != nullptr && p->next->data != value) {
p = p->next;
}
if (p->next != nullptr) {
Node* temp = p->next;
p->next = temp->next;
delete temp;
}
}
```
4. 查找节点
查找节点需要遍历链表,直到找到目标节点或到达链表尾部。
```
Node* searchNode(Node* head, int value) {
Node* p = head->next;
while (p != nullptr && p->data != value) {
p = p->next;
}
return p;
}
```
5. 显示链表
显示链表需要遍历链表,依次输出每个节点的数据。
```
void displayList(Node* head) {
Node* p = head->next;
while (p != nullptr) {
cout << p->data << " ";
p = p->next;
}
cout << endl;
}
```
6. 通讯录设计(应用)
通讯录可以使用单链表来实现,每个节点存储一个人的姓名和电话号码。具体实现过程如下:
```
// 通讯录节点结构体
struct Person {
string name; // 姓名
string phone; // 电话号码
Person* next; // 指针域
};
// 创建通讯录
Person* createAddressBook() {
Person* head = new Person;
head->next = nullptr;
Person* tail = head;
int n;
cin >> n;
for (int i = 0; i < n; i++) {
Person* person = new Person;
cin >> person->name >> person->phone;
person->next = nullptr;
tail->next = person;
tail = person;
}
return head;
}
// 插入联系人
void insertPerson(Person* head, string name, string phone) {
Person* person = new Person;
person->name = name;
person->phone = phone;
person->next = head->next;
head->next = person;
}
// 删除联系人
void deletePerson(Person* head, string name) {
Person* p = head;
while (p->next != nullptr && p->next->name != name) {
p = p->next;
}
if (p->next != nullptr) {
Person* temp = p->next;
p->next = temp->next;
delete temp;
}
}
// 查找联系人
Person* searchPerson(Person* head, string name) {
Person* p = head->next;
while (p != nullptr && p->name != name) {
p = p->next;
}
return p;
}
// 显示通讯录
void displayAddressBook(Person* head) {
Person* p = head->next;
while (p != nullptr) {
cout << p->name << "\t" << p->phone << endl;
p = p->next;
}
}
```
以上是单链表的基本操作及通讯录应用的实现思路,具体实现过程中还需要考虑一些细节问题,例如输入格式检查、异常处理等。希望能够对您有所帮助。
阅读全文
相关推荐


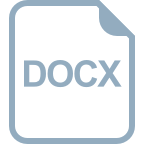
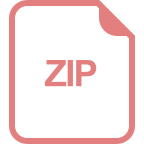
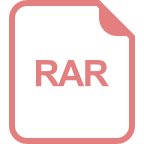
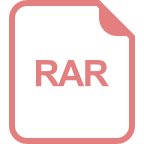
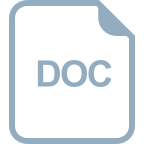
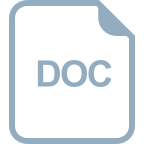
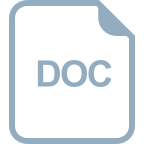
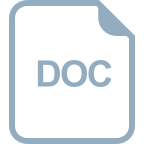
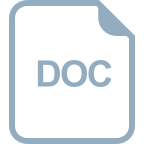
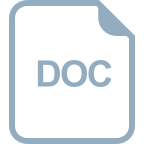




