分析此代码的运行过程public class Test { public static void main(String[] args) { System.out.println("main开始"); int x=0; try { x++; int y=x/0; x++; System.out.println(x); }catch(RuntimeException e){ System.out.println(e.getMessage()+"1"); }catch(Exception e){ System.out.println(e.getMessage()+"2"); }finally { x=x+2; } System.out.println(x); } }
时间: 2024-04-26 17:23:31 浏览: 20
这段代码主要是在 `main` 方法中进行的,具体运行过程如下:
1. 首先执行 `System.out.println("main开始");` 语句,输出 "main开始"。
2. 定义变量 `x` 并初始化为 0。
3. 执行 `try` 语句块中的语句,先执行 `x++`,此时 `x` 的值变为 1。
4. 执行 `int y=x/0;` 语句,由于除数为 0,会抛出一个 `ArithmeticException` 异常,直接跳转到 `catch` 语句块中。
5. 因为 `ArithmeticException` 是 `RuntimeException` 的子类,所以会匹配到第一个 `catch` 语句块,输出异常信息 "java.lang.ArithmeticException: / by zero1"。
6. 执行 `finally` 语句块中的语句,将 `x` 的值加 2,此时 `x` 的值变为 3。
7. 执行 `System.out.println(x);` 语句,输出 3。
因为除数为 0,导致抛出了异常,所以 `int y=x/0;` 语句后面的语句就不会执行了。由于有 `finally` 语句块,无论是否抛出异常,都会执行其中的语句。最终输出结果为 "main开始\njava.lang.ArithmeticException: / by zero1\n3"。
相关问题
class Test{ public static void main(String[] args){ System.out.println(i); int i =10; } }
这段代码会报错,因为变量 `i` 在使用之前被引用。在 `System.out.println(i)` 的位置,编译器会提示找不到符号 `i` 的错误。在 Java 中,变量必须先声明后使用。如果你想在 `System.out.println(i)` 中打印变量 `i` 的值,需要将其声明在使用之前。以下是修改后的代码:
```java
class Test {
public static void main(String[] args) {
int i = 10;
System.out.println(i);
}
}
```
这样修改后,代码就可以成功输出变量 `i` 的值 10。
class Test{ public static void main(String[] args){ { int i =10; } System.out.println(i); }
这段代码会报错,因为变量 `i` 的作用域仅限于内部的代码块中,无法在外部代码块中访问。在 `System.out.println(i)` 的位置,编译器会提示找不到符号 `i` 的错误。如果你想在外部访问变量 `i`,需要将其声明在外部代码块中。以下是修改后的代码:
```java
class Test {
public static void main(String[] args) {
int i;
{
i = 10;
}
System.out.println(i);
}
}
```
这样修改后,代码就可以成功输出变量 `i` 的值 10。
相关推荐
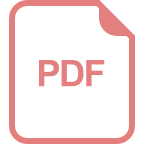
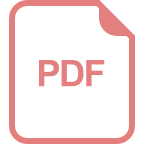
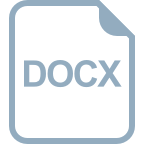













