public class Test { public static void main(String[] args) { int i = 0: Integer j = new Integer(0) ; System.out.println(i==j); System.out.println(j.equals(i));
时间: 2024-03-05 16:51:14 浏览: 269
这段代码存在语法错误,不能编译通过。其中第二行中冒号应该改为分号,变量类型应该为 `Integer` 而非 `int`。修改后的代码如下:
```
public class Test {
public static void main(String[] args) {
int i = 0;
Integer j = new Integer(0);
System.out.println(i == j);
System.out.println(j.equals(i));
}
}
```
这段代码首先定义了一个 `int` 类型的变量 `i`,并将其赋值为 `0`;然后定义了一个 `Integer` 类型的变量 `j`,并将其赋值为 `0` 的包装类型 `new Integer(0)`。接下来分别输出了 `i == j` 和 `j.equals(i)` 的结果。
由于 `i` 和 `j` 数据类型不同,因此在比较相等性时会进行类型转换。在 `i == j` 中,`j` 会被自动拆箱,得到 `0`,然后和 `i` 进行比较,因此结果为 `true`。在 `j.equals(i)` 中,由于 `i` 是一个 `int` 类型的基本数据类型,因此会自动装箱为 `Integer` 类型,然后进行比较,因此结果也为 `true`。
相关问题
public class Test { public static void main(String[] args) { int i = 0; Integer j = new Integer(0); System.out.println(i == j); System.out.println(j.equals(i)); }}
这段代码定义了一个类 `Test`,在其 `main` 方法中定义了一个 `int` 类型的变量 `i` 和一个 `Integer` 类型的变量 `j`,分别初始化为 `0` 和 `new Integer(0)`。接下来分别使用 `==` 和 `equals` 运算符比较 `i` 和 `j` 是否相等。
在 `i == j` 中,由于 `i` 是一个基本数据类型,而 `j` 是一个对象,因此会进行自动装箱操作,将 `i` 转换成 `Integer` 类型,然后再比较两个对象是否相等。由于 `Integer` 类型是一个对象,因此比较的是两个对象的引用地址,而不是对象的值。在这个例子中,由于 `i` 和 `j` 都是 `0`,因此会将它们拆箱成基本数据类型进行比较,结果为 `true`。
在 `j.equals(i)` 中,由于 `equals` 方法是一个对象方法,因此需要调用 `j` 对象的 `equals` 方法。由于 `j` 是一个 `Integer` 类型的对象,因此会调用 `Integer` 类中的 `equals` 方法。`equals` 方法会先判断参数是否为 `null` 或者和 `j` 对象类型不一致,如果满足这些条件则直接返回 `false`。否则会将参数转换成 `int` 类型,然后比较两个 `int` 类型的值是否相等。在这个例子中,由于 `i` 和 `j` 都是 `0`,因此它们的值相等,`equals` 方法会返回 `true`。
因此,这段代码会输出两行 `true`。
public class test { public static void main(String args[]) { Integer intObj=Integer.valueOf(args[args.length-1]); int i = intObj.intValue(); if(args.length > 1) System.out.println(i); if(args.length > 0) System.out.println(i - 1); else System.out.println(i - 2); } } 运行程序 , 输入如下命令: java test 2
运行程序后,会输出以下结果:
1
这是因为,输入的命令行参数只有一个,即 "2",所以 args.length 的值为 1,进入第一个 if 语句,输出 i 的值,即 2 的整数形式,为 2。
然后进入第二个 if 语句,也会输出 i - 1 的值,即 2 - 1,为 1。
因为 args.length 不大于 0,所以不会进入 else 语句。
阅读全文
相关推荐
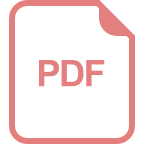
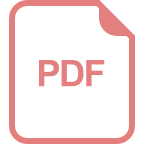












