c语言给定一个整数数组 nums和一个整数目标值 target,请你在该数组中找出 和为目标值 target 的那两个整数,并返回它们的数组下标。
时间: 2023-11-16 17:52:26 浏览: 40
在C语言中,可以使用哈希表来解决这个问题。首先,我们可以创建一个哈希表,将数组中的每个元素的值作为键,将其索引作为值,并遍历数组。在遍历过程中,我们可以通过查询哈希表来判断当前元素所需要的补数是否在数组中。如果存在,我们可以返回这两个数的索引。如果不存在,我们将当前元素的值和索引添加到哈希表中,以便后续的查询。
以下是实现该算法的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int val;
int index;
} HashNode;
int* twoSum(int* nums, int numsSize, int target, int* returnSize) {
int* result = (int*)malloc(2 * sizeof(int));
*returnSize = 0;
HashNode* hashTable = (HashNode*)malloc(numsSize * sizeof(HashNode));
for (int i = 0; i < numsSize; i++) {
hashTable[i].val = -1;
hashTable[i].index = -1;
}
for (int i = 0; i < numsSize; i++) {
int complement = target - nums[i];
int hash = (complement < 0 ? -complement : complement) % numsSize;
while (hashTable[hash].val != -1 && hashTable[hash].val != complement) {
hash = (hash + 1) % numsSize;
}
if (hashTable[hash].val != -1) {
result[0] = hashTable[hash].index;
result[1] = i;
*returnSize = 2;
break;
}
hashTable[hash].val = nums[i];
hashTable[hash].index = i;
}
free(hashTable);
return result;
}
int main() {
int nums[] = {2, 7, 11, 15};
int target = 9;
int returnSize;
int* result = twoSum(nums, sizeof(nums) / sizeof(nums[0]), target, &returnSize);
if (returnSize == 2) {
printf("The two numbers are at index %d and %d.\n", result[0], result[1]);
} else {
printf("No solution found.\n");
}
free(result);
return 0;
}
```
相关推荐
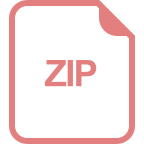
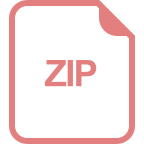
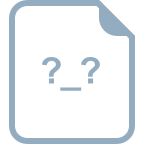














