python查找文件中关键字所在行
时间: 2024-10-10 19:12:47 浏览: 53
在Python中,可以使用内置函数`open()`打开文件,然后配合`readlines()`或逐行读取的方式来查找特定的关键字所在的行。这里有一个简单的示例:
```python
def find_keyword_in_file(filename, keyword):
with open(filename, 'r') as file:
lines = file.readlines() # 一次性读取所有行
for i, line in enumerate(lines, start=1): # 使用enumerate计数行号
if keyword in line:
print(f"Keyword '{keyword}' found at line {i}")
# 调用函数并传入需要搜索的文件名和关键字
find_keyword_in_file('yourfile.txt', 'your_search_keyword')
```
这个例子会打印出关键字在文件中首次出现的所有行号。如果你只想找到第一个匹配项,可以在循环结束后结束函数。
相关问题
python从txt中提取关键字所在行,并删除重复项后生成新的txt
可以使用Python中的set数据结构来实现该功能。以下是一个示例代码:
```python
# 定义关键字
keywords = ["apple", "banana", "orange"]
# 读取原始文本文件
with open("input.txt", "r") as f:
lines = f.readlines()
# 查找包含关键字的行
matched_lines = set()
for i, line in enumerate(lines):
if any(keyword in line for keyword in keywords):
matched_lines.add(i)
# 生成新的文本文件
with open("output.txt", "w") as f:
for i in matched_lines:
f.write(lines[i])
# 输出结果
print("提取并去重后的文本文件已生成。")
```
在这个示例中,我们首先定义了关键字列表。然后读取了原始文本文件,并使用enumerate函数获取每一行的行号和内容。接着,我们使用了一个for循环和any函数来查找包含关键字的行,并将其行号加入到一个set集合中去重。最后,我们使用了一个for循环和write函数将匹配的行写入到新的文本文件中。
需要注意的是,以上示例代码仅适用于小型文本文件。如果原始文件很大,可以考虑使用迭代器或者分块读取的方式来处理。
用python建立csv文件,输入数据,并输出某一个关键字所在行的所有数据
在Python中,你可以使用内置的`csv`模块来创建CSV文件、写入数据并读取特定关键字所在的行。以下是一个简单的步骤示例:
首先,你需要导入`csv`模块:
```python
import csv
```
然后,你可以选择创建一个新的CSV文件,如果文件不存在的话:
```python
# 创建文件,如果不存在则创建
with open('example.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
# 写入表头
writer.writerow(['Column1', 'Column2', 'Keyword Column']) # 假设关键字列名是'Keyword Column'
```
接着,你可以逐行写入数据,例如使用列表来存储每一行的数据:
```python
data_rows = [
['Value1', 'Value2', 'KeywordValue'], # 关键字值在这列
['AnotherValue1', 'AnotherValue2', 'KeywordValue'],
# 更多数据...
]
for row in data_rows:
writer.writerow(row)
```
最后,如果你想根据关键字查找并输出对应的行,可以这样操作:
```python
# 打开已有的文件以读模式
with open('example.csv', 'r') as csvfile:
reader = csv.reader(csvfile)
for row_num, row_data in enumerate(reader): # 遍历每一行
if 'KeywordValue' in row_data: # 检查关键字是否存在
print(f"找到关键字,第 {row_num+1} 行数据: {row_data}")
```
阅读全文
相关推荐
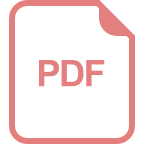
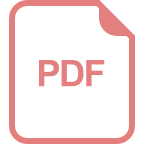
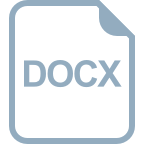
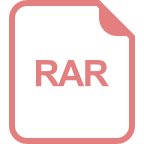
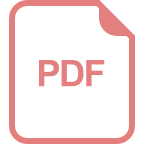
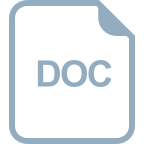
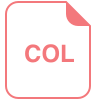
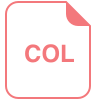
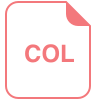
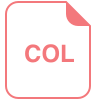
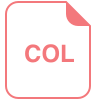
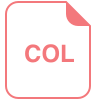



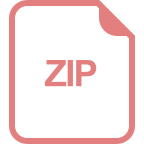