function checkYearLength() { const dateInput = document.getElementById("date"); const year = dateInput.value.substring(0, 4); if (year.length > 4) { const correctedValue = year.substring(0, 4) + dateInput.value.substring(4); dateInput.value = correctedValue; } }有bug,判断不了有没有超过4个
时间: 2024-03-05 09:49:24 浏览: 21
这个函数的问题在于它只检查了字符串的前四个字符,而不是整个字符串。所以,当输入的年份超过4位数时,它就会把整个字符串截断,只保留前四个字符。这个问题可以通过将 year.length 改为 dateInput.value.length 来解决。修改后的代码如下:
```
function checkYearLength() {
const dateInput = document.getElementById("date");
const year = dateInput.value.substring(0, 4);
if (dateInput.value.length > 4) {
const correctedValue = year.substring(0, 4) + dateInput.value.substring(4);
dateInput.value = correctedValue;
}
}
```
这样,当输入的字符串长度超过4时,函数就会把整个字符串保留下来,而不是截断。
相关问题
const canvas = document.getElementById('myCanvas'); const ctx = canvas.getContext('2d'); const text = '这是一段需要换行的文字,第一行要顶格2个字符'; const maxWidth = 200; const lineHeight = 30; const x = 10; let y = 50; // 绘制第一行文字 ctx.fillText(text.substring(0, 2), x, y); // 计算第一行文字的宽度 const firstLineWidth = ctx.measureText(text.substring(0, 2)).width; // 绘制剩余部分的文字 let index = 2; while (index < text.length) { const line = getNextLine(text, index, maxWidth - firstLineWidth); ctx.fillText(line, x + firstLineWidth, y); y += lineHeight; index += line.length; } // 获取下一行要绘制的文字 function getNextLine(text, startIndex, maxWidth) { let endIndex = startIndex; let lineWidth = 0; while (endIndex < text.length && lineWidth < maxWidth) { lineWidth += ctx.measureText(text.charAt(endIndex)).width; endIndex++; } // 如果到达了最后一个字符仍然小于最大宽度,则直接返回 if (endIndex === text.length && lineWidth <= maxWidth) { return text.substring(startIndex); } // 否则返回到上一个空格处 for (let i = endIndex - 1; i > startIndex; i--) { if (text.charAt(i) === ' ') { endIndex = i + 1; break; } } return text.substring(startIndex, endIndex); }给这段代码增加行数显示,超出限制行数用...显示
可以在绘制文字之前增加一个计数器,每绘制一行文字就将计数器减1,当计数器为0时,即达到了限制行数,就不再绘制文字。同时,需要在最后一行文字结尾处加上省略号,以表示该行文字被截断了。
修改后的代码如下:
```
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
const text = '这是一段需要换行的文字,第一行要顶格2个字符';
const maxWidth = 200;
const lineHeight = 30;
const x = 10;
let y = 50;
let lineCount = 3; // 限制行数为3行
// 绘制第一行文字
ctx.fillText(text.substring(0, 2), x, y);
// 计算第一行文字的宽度
const firstLineWidth = ctx.measureText(text.substring(0, 2)).width;
// 绘制剩余部分的文字
let index = 2;
while (index < text.length && lineCount > 0) {
const line = getNextLine(text, index, maxWidth - firstLineWidth);
// 绘制当前行文字
ctx.fillText(line, x + firstLineWidth, y);
// 更新纵坐标和行数计数器
y += lineHeight;
lineCount--;
// 更新字符索引
index += line.length;
}
// 如果还有剩余的文字未绘制,则在最后一行结尾处加上省略号
if (index < text.length) {
const lastLine = '...' + text.substring(index);
const lastLineWidth = ctx.measureText(lastLine).width;
ctx.fillText(lastLine, x + firstLineWidth, y);
}
// 获取下一行要绘制的文字
function getNextLine(text, startIndex, maxWidth) {
let endIndex = startIndex;
let lineWidth = 0;
while (endIndex < text.length && lineWidth < maxWidth) {
lineWidth += ctx.measureText(text.charAt(endIndex)).width;
endIndex++;
}
// 如果到达了最后一个字符仍然小于最大宽度,则直接返回
if (endIndex === text.length && lineWidth <= maxWidth) {
return text.substring(startIndex);
}
// 否则返回到上一个空格处
for (let i = endIndex - 1; i > startIndex; i--) {
if (text.charAt(i) === ' ') {
endIndex = i + 1;
break;
}
}
return text.substring(startIndex, endIndex);
}
```
在上述代码中,我们加入了一个 lineCount 变量,用于记录还可以绘制的行数。每绘制一行文字,就将 lineCount 减1,当 lineCount 为0时,即达到了限制行数,就不再绘制文字。同时,如果还有剩余的文字未绘制,则在最后一行结尾处加上省略号。
HTML5 中的 date 输入,年份会出现6位数,如何限制。
HTML5 中的 date 输入类型是支持显示6位数年份的,例如 "yyyyyy-mm-dd" 的格式。如果你想限制年份为4位数,可以使用 JavaScript 进行限制。
以下是一个示例代码,可以限制年份为 4 位数:
```html
<label for="date">选择日期:</label>
<input type="date" id="date" name="date" onchange="checkYearLength()">
<script>
function checkYearLength() {
const dateInput = document.getElementById("date");
const year = dateInput.value.substring(0, 4);
if (year.length > 4) {
const correctedValue = year.substring(0, 4) + dateInput.value.substring(4);
dateInput.value = correctedValue;
}
}
</script>
```
在上面的代码中,我们给 date 输入框添加了一个 onchange 事件,当用户选择日期时,会触发 checkYearLength 函数。该函数会获取用户选择的日期,并检查年份的长度是否大于 4。如果是,就会截取前 4 位作为年份,并将日期重新设置为正确的格式。
这样,用户就无法输入 6 位数的年份了。
相关推荐
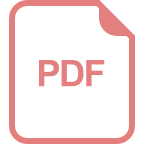
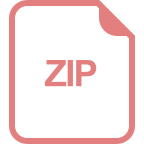












