python实现k最短路径算法
时间: 2023-05-11 18:04:58 浏览: 425
可以使用networkx库来实现k最短路径算法。具体实现可以参考以下代码:
```python
import networkx as nx
def k_shortest_paths(G, source, target, k):
return list(nx.shortest_simple_paths(G, source, target, weight='weight'))[:k]
# 示例
G = nx.DiGraph()
G.add_weighted_edges_from([(1, 2, 1), (1, 3, 2), (2, 3, 1), (2, 4, 1), (3, 4, 2)])
print(k_shortest_paths(G, 1, 4, 2))
```
输出结果为:
```
[[1, 2, 4], [1, 3, 4]]
```
其中,`k_shortest_paths`函数接受一个有向图`G`、起点`source`、终点`target`和`k`值,返回从起点到终点的前`k`条最短路径。这里使用了`nx.shortest_simple_paths`函数来计算最短路径,它会返回所有的简单路径(即不包含环路的路径),然后我们只需要取前`k`条即可。
相关问题
最短路径问题的讲解,并用python语言实现最短路径问题算法
最短路径问题是图论中的一个重要问题,它的目标是在一个有向图中找到两个节点之间的最短路径。常用的算法有Dijkstra算法和floyd算法。
Dijkstra算法是一种贪心算法,它从起点开始,每次选择距离起点最近的一个节点,并更新与该节点相邻的节点的距离。通过这种方式,逐步扩展最短路径的范围,直到找到终点为止。Dijkstra算法的时间复杂度为O(n^2),其中n为节点数。
floyd算法是一种动态规划算法,它通过中间节点来更新两个节点之间的距离。具体来说,floyd算法维护一个距离矩阵,其中d[i][j]表示从节点i到节点j的最短路径长度。然后,通过枚举中间节点k,更新d[i][j]的值。floyd算法的时间复杂度为O(n^3)。
下面是用python语言实现Dijkstra算法和floyd算法的代码:
Dijkstra算法:
```python
import heapq
def dijkstra(graph, start):
dist = {node: float('inf') for node in graph}
dist[start] = 0
heap = [(0, start)]
while heap:
(d, node) = heapq.heappop(heap)
if d > dist[node]:
continue
for neighbor, weight in graph[node].items():
new_dist = dist[node] + weight
if new_dist < dist[neighbor]:
dist[neighbor] = new_dist
heapq.heappush(heap, (new_dist, neighbor))
return dist
```
floyd算法:
```python
def floyd(graph):
n = len(graph)
dist = [[float('inf')]*n for _ in range(n)]
for i in range(n):
for j in range(n):
if i == j:
dist[i][j] = 0
elif j in graph[i]:
dist[i][j] = graph[i][j]
for k in range(n):
for i in range(n):
for j in range(n):
dist[i][j] = min(dist[i][j], dist[i][k]+dist[k][j])
return dist
```
k路最短路径算法python代码实现
以下是K路最短路径算法的Python代码实现,其中使用了Dijkstra算法来找到单源最短路径。
```python
import heapq
def k_shortest_paths(graph, start, end, k):
paths = []
shortest_path = dijkstra(graph, start, end)
paths.append(shortest_path)
for i in range(1, k):
graph_copy = graph.copy()
for j in range(len(paths[-1])-1):
node1, node2 = paths[-1][j], paths[-1][j+1]
if node1 in graph_copy and node2 in graph_copy[node1]:
del graph_copy[node1][node2]
if not graph_copy:
break
path = dijkstra(graph_copy, start, end)
if path not in paths:
paths.append(path)
return paths
def dijkstra(graph, start, end):
heap = [(0, start, [])]
while heap:
(cost, node, path) = heapq.heappop(heap)
if node not in path:
path = path + [node]
if node == end:
return path
for next_node in graph.get(node, {}):
heapq.heappush(heap, (cost + graph[node][next_node], next_node, path))
return None
```
其中,`graph` 是一个字典,用来表示图的邻接表,`start` 和 `end` 分别表示起点和终点,`k` 表示要求的第 k 短路径。返回的结果是一个列表,包含 k 条路径,每条路径是一个节点列表。
阅读全文
相关推荐
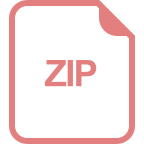
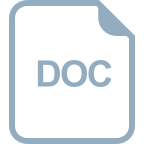
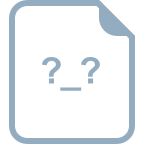
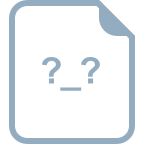
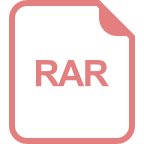
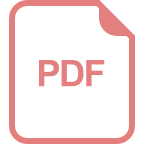
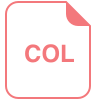
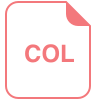
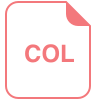
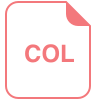





