设计一个图书管理系统详细过程要有代码
时间: 2024-03-10 07:47:11 浏览: 109
好的,下面是一个简单的图书管理系统的设计和实现,包含C#代码和SQL Server数据库的建表语句,仅供参考:
1. 数据库设计
创建数据库:BookManagementSystem
创建用户表:User
```sql
CREATE TABLE [dbo].[User](
[Id] [int] IDENTITY(1,1) NOT NULL,
[Username] [nvarchar](50) NOT NULL,
[Password] [nvarchar](50) NOT NULL,
[Email] [nvarchar](50) NULL,
[Role] [int] NOT NULL,
CONSTRAINT [PK_User] PRIMARY KEY CLUSTERED
(
[Id] ASC
)
)
```
其中,Role字段表示用户角色,0表示普通用户,1表示管理员。
创建图书表:Book
```sql
CREATE TABLE [dbo].[Book](
[Id] [int] IDENTITY(1,1) NOT NULL,
[Title] [nvarchar](50) NOT NULL,
[Author] [nvarchar](50) NOT NULL,
[Publisher] [nvarchar](50) NOT NULL,
[ISBN] [nvarchar](50) NOT NULL,
[Price] [money] NOT NULL,
[Category] [nvarchar](50) NOT NULL,
[Stock] [int] NOT NULL,
CONSTRAINT [PK_Book] PRIMARY KEY CLUSTERED
(
[Id] ASC
)
)
```
创建借阅记录表:BorrowRecord
```sql
CREATE TABLE [dbo].[BorrowRecord](
[Id] [int] IDENTITY(1,1) NOT NULL,
[BookId] [int] NOT NULL,
[UserId] [int] NOT NULL,
[BorrowTime] [datetime] NOT NULL,
[ReturnTime] [datetime] NULL,
[Status] [int] NOT NULL,
CONSTRAINT [PK_BorrowRecord] PRIMARY KEY CLUSTERED
(
[Id] ASC
)
)
```
其中,Status字段表示借阅状态,0表示已借未还,1表示已还。
2. 界面设计
使用Windows Forms实现图书管理系统界面,包含登录、注册、图书管理、借阅管理、数据统计等模块。
3. 功能实现
(1)用户管理模块
用户注册:
```csharp
private void btnRegister_Click(object sender, EventArgs e)
{
string username = txtUsername.Text.Trim();
string password = txtPassword.Text.Trim();
string email = txtEmail.Text.Trim();
int role = 0; // 普通用户
if (string.IsNullOrEmpty(username) || string.IsNullOrEmpty(password))
{
MessageBox.Show("用户名或密码不能为空!");
return;
}
// 判断用户名是否已存在
if (UserService.GetUserByUsername(username) != null)
{
MessageBox.Show("该用户名已被注册!");
return;
}
User user = new User
{
Username = username,
Password = Common.MD5(password),
Email = email,
Role = role
};
if (UserService.AddUser(user))
{
MessageBox.Show("注册成功!");
this.Close();
}
else
{
MessageBox.Show("注册失败,请稍后再试!");
}
}
```
用户登录:
```csharp
private void btnLogin_Click(object sender, EventArgs e)
{
string username = txtUsername.Text.Trim();
string password = txtPassword.Text.Trim();
if (string.IsNullOrEmpty(username) || string.IsNullOrEmpty(password))
{
MessageBox.Show("用户名或密码不能为空!");
return;
}
User user = UserService.GetUserByUsername(username);
if (user == null)
{
MessageBox.Show("该用户不存在!");
return;
}
if (user.Password.Equals(Common.MD5(password)))
{
MessageBox.Show("登录成功!");
this.Hide();
MainForm mainForm = new MainForm(user);
mainForm.ShowDialog();
this.Close();
}
else
{
MessageBox.Show("密码错误!");
}
}
```
(2)图书管理模块
添加图书:
```csharp
private void btnAdd_Click(object sender, EventArgs e)
{
string title = txtTitle.Text.Trim();
string author = txtAuthor.Text.Trim();
string publisher = txtPublisher.Text.Trim();
string isbn = txtISBN.Text.Trim();
decimal price = decimal.Parse(txtPrice.Text.Trim());
string category = txtCategory.Text.Trim();
int stock = int.Parse(txtStock.Text.Trim());
Book book = new Book
{
Title = title,
Author = author,
Publisher = publisher,
ISBN = isbn,
Price = price,
Category = category,
Stock = stock
};
if (BookService.AddBook(book))
{
MessageBox.Show("添加成功!");
LoadBooks();
}
else
{
MessageBox.Show("添加失败,请稍后再试!");
}
}
```
查看图书:
```csharp
private void dgvBooks_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (e.RowIndex < 0 || e.RowIndex >= dgvBooks.Rows.Count - 1)
{
return;
}
int bookId = int.Parse(dgvBooks.Rows[e.RowIndex].Cells["Id"].Value.ToString());
Book book = BookService.GetBookById(bookId);
if (book != null)
{
txtTitle.Text = book.Title;
txtAuthor.Text = book.Author;
txtPublisher.Text = book.Publisher;
txtISBN.Text = book.ISBN;
txtPrice.Text = book.Price.ToString();
txtCategory.Text = book.Category;
txtStock.Text = book.Stock.ToString();
}
}
```
修改图书:
```csharp
private void btnModify_Click(object sender, EventArgs e)
{
int bookId = int.Parse(dgvBooks.SelectedRows[0].Cells["Id"].Value.ToString());
string title = txtTitle.Text.Trim();
string author = txtAuthor.Text.Trim();
string publisher = txtPublisher.Text.Trim();
string isbn = txtISBN.Text.Trim();
decimal price = decimal.Parse(txtPrice.Text.Trim());
string category = txtCategory.Text.Trim();
int stock = int.Parse(txtStock.Text.Trim());
Book book = new Book
{
Id = bookId,
Title = title,
Author = author,
Publisher = publisher,
ISBN = isbn,
Price = price,
Category = category,
Stock = stock
};
if (BookService.UpdateBook(book))
{
MessageBox.Show("修改成功!");
LoadBooks();
}
else
{
MessageBox.Show("修改失败,请稍后再试!");
}
}
```
删除图书:
```csharp
private void btnDelete_Click(object sender, EventArgs e)
{
int bookId = int.Parse(dgvBooks.SelectedRows[0].Cells["Id"].Value.ToString());
if (BookService.DeleteBook(bookId))
{
MessageBox.Show("删除成功!");
LoadBooks();
}
else
{
MessageBox.Show("删除失败,请稍后再试!");
}
}
```
(3)借阅管理模块
借阅图书:
```csharp
private void btnBorrow_Click(object sender, EventArgs e)
{
int bookId = int.Parse(dgvBooks.SelectedRows[0].Cells["Id"].Value.ToString());
int userId = _user.Id;
DateTime borrowTime = DateTime.Now;
BorrowRecord borrowRecord = new BorrowRecord
{
BookId = bookId,
UserId = userId,
BorrowTime = borrowTime,
ReturnTime = null,
Status = 0 // 已借未还
};
if (BorrowRecordService.AddBorrowRecord(borrowRecord))
{
MessageBox.Show("借阅成功!");
LoadBorrowRecords();
}
else
{
MessageBox.Show("借阅失败,请稍后再试!");
}
}
```
归还图书:
```csharp
private void btnReturn_Click(object sender, EventArgs e)
{
int borrowRecordId = int.Parse(dgvBorrowRecords.SelectedRows[0].Cells["Id"].Value.ToString());
DateTime returnTime = DateTime.Now;
if (BorrowRecordService.ReturnBook(borrowRecordId, returnTime))
{
MessageBox.Show("归还成功!");
LoadBorrowRecords();
}
else
{
MessageBox.Show("归还失败,请稍后再试!");
}
}
```
(4)数据统计模块
查询图书借阅量:
```csharp
private void btnQuery_Click(object sender, EventArgs e)
{
string category = txtCategory.Text.Trim();
int borrowCount = 0;
List<Book> books = BookService.GetBooksByCategory(category);
foreach (Book book in books)
{
borrowCount += BorrowRecordService.GetBorrowCountByBookId(book.Id);
}
MessageBox.Show($"该分类图书的借阅量为:{borrowCount}");
}
```
(5)系统设置模块
修改借阅期限:
```csharp
private void btnSave_Click(object sender, EventArgs e)
{
int days = int.Parse(txtDays.Text.Trim());
if (SettingService.UpdateSetting("BorrowDays", days.ToString()))
{
MessageBox.Show("保存成功!");
}
else
{
MessageBox.Show("保存失败,请稍后再试!");
}
}
```
4. 总结
上述代码仅为示例,具体实现方式可能因需求而异。在实际开发中,还需要考虑安全性、性能、可扩展性等方面的问题,如防止SQL注入、使用缓存技术、使用分布式架构等。
阅读全文
相关推荐
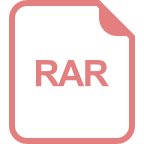
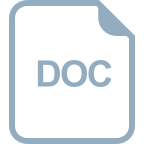
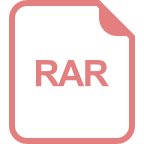
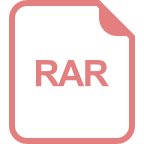
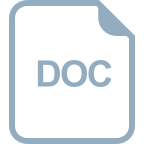
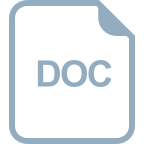
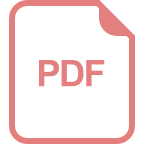
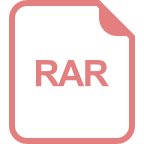
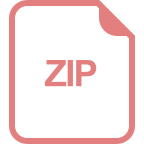
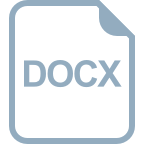
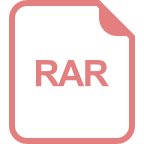
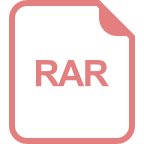
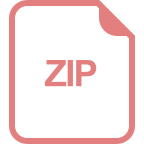
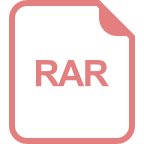
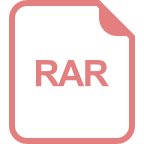
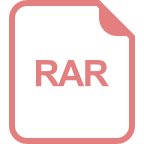