STM32驱动vga显示彩条程序
时间: 2024-02-01 11:02:56 浏览: 20
以下是一个简单的基于STM32的VGA显示彩条程序,可以让你开始学习如何驱动VGA显示器。
```c
#include "stm32f10x.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_rcc.h"
#include "stm32f10x_tim.h"
#define PIXELS_PER_LINE 800
#define PIXEL_CLOCK 25175000
#define VH_SYNC_WIDTH 96
#define VH_BACK_PORCH 48
#define VH_FRONT_PORCH 16
volatile uint16_t framebuffer[PIXELS_PER_LINE];
void init_vga_gpio(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_14 | GPIO_Pin_15;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_8 | GPIO_Pin_9 | GPIO_Pin_10 |
GPIO_Pin_11 | GPIO_Pin_12 | GPIO_Pin_13;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void init_vga_timer(void)
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_TimeBaseStructure.TIM_Prescaler = 0;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseStructure.TIM_Period = PIXELS_PER_LINE + VH_SYNC_WIDTH +
VH_BACK_PORCH + VH_FRONT_PORCH;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
}
void init_vga_timer_interrupt(void)
{
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_InitStructure.NVIC_IRQChannel = TIM2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 2;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
TIM_ITConfig(TIM2, TIM_IT_Update, ENABLE);
}
void TIM2_IRQHandler(void)
{
static uint16_t line_counter = 0;
static uint16_t pixel_counter = 0;
static uint16_t color_counter = 0;
static uint16_t red = 0;
static uint16_t green = 0;
static uint16_t blue = 0;
if (TIM_GetITStatus(TIM2, TIM_IT_Update) != RESET) {
TIM_ClearITPendingBit(TIM2, TIM_IT_Update);
if (line_counter < 480) {
if (pixel_counter < PIXELS_PER_LINE) {
if (color_counter < 16) {
framebuffer[pixel_counter] = ((red & 0x1F) << 11) |
((green & 0x3F) << 5) |
(blue & 0x1F);
color_counter++;
} else {
color_counter = 0;
blue += 2;
if (blue > 31) {
blue = 0;
green += 4;
if (green > 63) {
green = 0;
red += 2;
}
}
}
pixel_counter++;
} else {
pixel_counter = 0;
line_counter++;
}
} else {
line_counter = 0;
red = 0;
green = 0;
blue = 0;
}
TIM_SetCompare1(TIM2, pixel_counter + VH_SYNC_WIDTH +
VH_BACK_PORCH + VH_FRONT_PORCH);
}
}
int main(void)
{
init_vga_gpio();
init_vga_timer();
init_vga_timer_interrupt();
TIM_Cmd(TIM2, ENABLE);
while (1) {
}
}
```
这个程序使用了STM32的定时器和GPIO模块来生成VGA信号,并在显示器上显示彩条。程序中使用的是800x480像素的分辨率,每个像素使用16位颜色表示。在每个像素时钟周期中,程序会将当前颜色值写入framebuffer中,因此可以实现彩条的效果。
相关推荐
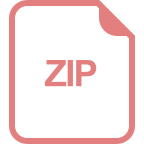
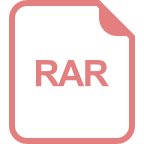














