excel_paths=[os.path.join(excel_dir,f)for f in os.listdir(excel_dir)if f.endswith('.xlsx')]修改這段代碼,需要找xlsx和xls,格式的文件
时间: 2024-04-08 21:29:13 浏览: 72
如果你想修改这段代码来同时查找.xlsx和.xls格式的文件,你可以使用以下代码:
```python
import os
excel_paths = [os.path.join(excel_dir, f) for f in os.listdir(excel_dir) if f.endswith(('.xlsx', '.xls'))]
```
在上述代码中,我们使用了`.endswith(('.xlsx', '.xls'))`来检查文件名是否以.xlsx或.xls结尾。如果是其中之一,就将文件路径添加到`excel_paths`列表中。这样,你就可以找到目录中同时包含.xlsx和.xls格式的文件。
相关问题
excel_dir=path excel_paths = [os.path.join(excel_dir, f) for f in os.listdir(excel_dir) if f.endswith(('.xlsx', '.xls'))] dfs=[] for excel_path in excel_paths: df=pd.read_excel(excel_path) dfs.append(df) merged_df=pd.concat(dfs) output_path=r"D:\SMT本部出勤匯總\系統人力.xlsx" merged_df.to_excel(output_path,index=False) #刪除路徑下文件 folder_path=path for filename in os.listdir(folder_path): file_path=os.path.join(folder_path,filename)#文件路徑 if os.path.isfile(file_path):#判斷是否為文件 os.remove(file_path)#刪除文件是這個代碼報的錯誤
这个错误通常表示在尝试删除文件时,文件路径可能无效或文件正在被其他程序使用。
我注意到你在代码中使用了变量`path`作为文件夹路径,但你没有给出`path`的具体值。请确保`path`变量包含正确的文件夹路径。
此外,还有一种可能性是某些文件正在被其他程序打开或使用,导致无法删除。你可以尝试在删除文件之前,先将文件关闭或确保没有其他程序正在使用它。可以尝试使用`try-except`块来捕捉可能的异常并输出错误信息,以便更好地理解问题所在。
以下是修改后的代码示例:
```python
import os
excel_dir = "path"
excel_paths = [os.path.join(excel_dir, f) for f in os.listdir(excel_dir) if f.endswith(('.xlsx', '.xls'))]
dfs = []
for excel_path in excel_paths:
df = pd.read_excel(excel_path)
dfs.append(df)
merged_df = pd.concat(dfs)
output_path = r"D:\SMT本部出勤匯總\系統人力.xlsx"
merged_df.to_excel(output_path, index=False)
# 删除路径下的文件
folder_path = "path"
for filename in os.listdir(folder_path):
file_path = os.path.join(folder_path, filename) # 文件路径
try:
if os.path.isfile(file_path): # 判断是否为文件
os.remove(file_path) # 删除文件
except Exception as e:
print(f"删除文件发生错误: {e}")
```
请确保将`path`替换为实际的文件夹路径。如果问题仍然存在,请提供完整的错误消息以供进一步调试。
excel_dir=path excel_paths=[os.path.join(excel_dir,f)for f in os.listdir(excel_dir)if f.endswith('.xlsx')] dfs=[] for excel_path in excel_paths: df=pd.read_excel(excel_path) dfs.append(df) merged_df=pd.concat(dfs) output_path=r"D:\SMT本部出勤匯總\出勤資料.xlsx" merged_df.to_excel(output_path,index=False) #刪除路徑下文件 folder_path=path for filename in os.listdir(folder_path): file_path=os.path.join(folder_path,filename)#文件路徑 if os.path.isfile(file_path):#判斷是否為文件 os.remove(file_path)#刪除文件 添加一個條件,這個運行完畢后才繼續運行下面的程序
要在删除文件后添加一个条件,确保该操作完成后再继续执行其他程序,你可以使用`try-except`块来捕捉可能的异常并输出错误信息。
以下是修改后的代码示例:
```python
import os
excel_dir = "path"
excel_paths = [os.path.join(excel_dir, f) for f in os.listdir(excel_dir) if f.endswith('.xlsx')]
dfs = []
for excel_path in excel_paths:
df = pd.read_excel(excel_path)
dfs.append(df)
merged_df = pd.concat(dfs)
output_path = r"D:\SMT本部出勤匯總\出勤資料.xlsx"
merged_df.to_excel(output_path, index=False)
# 删除路径下的文件
folder_path = "path"
for filename in os.listdir(folder_path):
file_path = os.path.join(folder_path, filename) # 文件路径
try:
if os.path.isfile(file_path): # 判断是否为文件
os.remove(file_path) # 删除文件
except Exception as e:
print(f"删除文件发生错误: {e}")
# 在这里继续执行其他程序
```
请确保将`path`替换为实际的文件夹路径。如果删除文件时发生错误,将会打印错误信息,并继续执行其他程序。
阅读全文
相关推荐
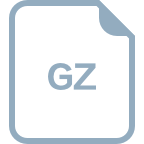


path = 'D:/SMT本部出勤' excel_dir = path excel_paths = [os.path.join(excel_dir, f) for f in os.listdir(excel_dir) if f.endswith('.xls')] dfs = [] for excel_path in excel_paths: df = pd.read_excel(excel_path, engine='openpyxl') df = df.replace({'<a href=': '', '
': ''}, regex=True) # 去除超链接标签 dfs.append(df) merged_df = pd.concat(dfs) output_path = r"D:\SMT本部出勤匯總\熱資料.xlsx" merged_df.to_excel(output_path, index=False),zipfile.BadZipFile: File is not a zip file報這個











