2、编写一个学生和教师数据输入和显示程序。其中,学生数据有编号、姓名、班级和成绩,教师数据有编号、姓名、职称和部门。 要求: (1)将编号、姓名输入和显示设计成一个类Person; (2)设计类Person的派生类:学生类Student和教师类Teacher; (3)各个类的声明放在相应的头文件中(*.h),类的实现放在相应的实现文件中(*.cpp):person.h,person.cpp,student.h,student.cpp,teacher.h,teacher.cpp; (4)编写一个主文件(SY
时间: 2023-05-29 18:03:44 浏览: 179
STEM.cpp),包含主函数main(),程序从键盘输入学生和教师数据,将其存入相应的对象中,然后输出显示。程序运行示例如下:
输入教师数据:
编号 姓名 职称 部门
1001 张三 教授 计算机学院
1002 李四 副教授 电子工程学院
输入学生数据:
编号 姓名 班级 成绩
2011001 王五 1班 80
2011002 赵六 2班 75
输出教师数据:
编号 姓名 职称 部门
1001 张三 教授 计算机学院
1002 李四 副教授 电子工程学院
输出学生数据:
编号 姓名 班级 成绩
2011001 王五 1班 80
2011002 赵六 2班 75
【参考答案】
【person.h】
```cpp
#ifndef PERSON_H
#define PERSON_H
#include <string>
using namespace std;
class Person {
public:
Person();
Person(int id, string name);
void setId(int id);
int getId();
void setName(string name);
string getName();
virtual void display(); //虚函数
private:
int id;
string name;
};
#endif
```
【person.cpp】
```cpp
#include "person.h"
#include <iostream>
using namespace std;
Person::Person() {}
Person::Person(int id, string name) {
this->id = id;
this->name = name;
}
void Person::setId(int id) {
this->id = id;
}
int Person::getId() {
return id;
}
void Person::setName(string name) {
this->name = name;
}
string Person::getName() {
return name;
}
void Person::display() {
cout << "编号:" << id << ",姓名:" << name << "。" << endl;
}
```
【student.h】
```cpp
#ifndef STUDENT_H
#define STUDENT_H
#include "person.h"
#include <string>
using namespace std;
class Student : public Person {
public:
Student();
Student(int id, string name, string cls, int score);
void setCls(string cls);
string getCls();
void setScore(int score);
int getScore();
virtual void display(); //重写虚函数
private:
string cls;
int score;
};
#endif
```
【student.cpp】
```cpp
#include "student.h"
#include <iostream>
using namespace std;
Student::Student() {}
Student::Student(int id, string name, string cls, int score) : Person(id, name) {
this->cls = cls;
this->score = score;
}
void Student::setCls(string cls) {
this->cls = cls;
}
string Student::getCls() {
return cls;
}
void Student::setScore(int score) {
this->score = score;
}
int Student::getScore() {
return score;
}
void Student::display() {
cout << "编号:" << getId() << ",姓名:" << getName() << ",班级:" << cls << ",成绩:" << score << "。" << endl;
}
```
【teacher.h】
```cpp
#ifndef TEACHER_H
#define TEACHER_H
#include "person.h"
#include <string>
using namespace std;
class Teacher : public Person {
public:
Teacher();
Teacher(int id, string name, string title, string depart);
void setTitle(string title);
string getTitle();
void setDepart(string depart);
string getDepart();
virtual void display(); //重写虚函数
private:
string title;
string depart;
};
#endif
```
【teacher.cpp】
```cpp
#include "teacher.h"
#include <iostream>
using namespace std;
Teacher::Teacher() {}
Teacher::Teacher(int id, string name, string title, string depart) : Person(id, name) {
this->title = title;
this->depart = depart;
}
void Teacher::setTitle(string title) {
this->title = title;
}
string Teacher::getTitle() {
return title;
}
void Teacher::setDepart(string depart) {
this->depart = depart;
}
string Teacher::getDepart() {
return depart;
}
void Teacher::display() {
cout << "编号:" << getId() << ",姓名:" << getName() << ",职称:" << title << ",部门:" << depart << "。" << endl;
}
```
【STEM.cpp】
```cpp
#include "person.h"
#include "student.h"
#include "teacher.h"
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<Person*> persons; //定义Person类型指针的向量
int id, score;
string name, cls, title, depart;
//输入教师数据
cout << "输入教师数据:" << endl;
while (cin >> id >> name >> title >> depart) {
Teacher* t = new Teacher(id, name, title, depart); //创建教师对象
persons.push_back(t); //将教师对象指针添加到向量中
}
//清空输入缓冲区
cin.clear();
cin.sync();
//输入学生数据
cout << "输入学生数据:" << endl;
while (cin >> id >> name >> cls >> score) {
Student* s = new Student(id, name, cls, score); //创建学生对象
persons.push_back(s); //将学生对象指针添加到向量中
}
//输出教师数据
cout << "输出教师数据:" << endl;
for (int i = 0; i < persons.size(); i++) {
Teacher* t = dynamic_cast<Teacher*>(persons[i]); //将Person类型转换为Teacher类型
if (t != NULL) { //如果转换成功
t->display(); //调用Teacher类的display()函数
}
}
//输出学生数据
cout << "输出学生数据:" << endl;
for (int i = 0; i < persons.size(); i++) {
Student* s = dynamic_cast<Student*>(persons[i]); //将Person类型转换为Student类型
if (s != NULL) { //如果转换成功
s->display(); //调用Student类的display()函数
}
}
//释放内存
for (int i = 0; i < persons.size(); i++) {
delete persons[i];
}
persons.clear();
return 0;
}
```
阅读全文
相关推荐
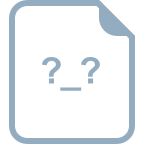














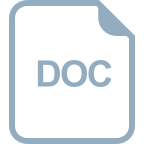
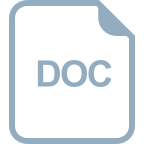
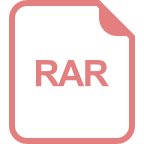