Unity 人物通过WASD移动代码
时间: 2024-06-10 14:08:30 浏览: 8
如下:
```
public float moveSpeed = 5f;
void Update()
{
float horizontalInput = Input.GetAxis("Horizontal");
float verticalInput = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(horizontalInput, 0f, verticalInput) * moveSpeed * Time.deltaTime;
transform.position += movement;
}
```
请注意,以上代码只是示例代码,您需要根据具体情况进行修改以适应您的项目。
相关问题
unity3d人物加速移动代码
在Unity3D中,创建一个角色加速移动通常涉及到使用Character Controller组件,因为它是专门设计用于处理2D或3D游戏中的角色移动的。以下是一个简单的示例代码,展示如何实现角色的基础移动和加速:
```csharp
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float speed = 5.0f; // 角色移动速度
private CharacterController cc; // 角色控制器
void Start()
{
cc = GetComponent<CharacterController>();
}
void Update()
{
// 获取玩家输入(假设Input.GetAxis是获取方向键输入)
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
// 创建一个Vector3对象来存储移动方向
Vector3 movement = new Vector3(moveHorizontal, 0, moveVertical);
// 如果按下W或S,则增加或减少角色的速度
if (moveVertical > 0)
{
speed = Mathf.Lerp(speed, speed + 1.0f * Time.deltaTime, 0.1f); // 加速
}
else if (moveVertical < 0)
{
speed = Mathf.Lerp(speed, speed - 1.0f * Time.deltaTime, 0.1f); // 减速
}
// 使用CharacterController的Move方法,以当前速度和移动方向移动角色
cc.SimpleMove(movement * speed);
}
}
```
unity人物旋转移动代码
以下是一个简单的 Unity 人物旋转移动代码示例:
```csharp
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float moveSpeed = 5f;
public float turnSpeed = 10f;
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void FixedUpdate()
{
float horizontal = Input.GetAxis("Horizontal");
float vertical = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(horizontal, 0f, vertical) * moveSpeed * Time.fixedDeltaTime;
rb.MovePosition(transform.position + transform.TransformDirection(movement));
if (movement.magnitude > 0f)
{
Quaternion targetRotation = Quaternion.LookRotation(movement.normalized);
rb.MoveRotation(Quaternion.Lerp(rb.rotation, targetRotation, turnSpeed * Time.fixedDeltaTime));
}
}
}
```
这个代码包含以下功能:
- 控制人物向前、后、左、右移动。
- 控制人物随着移动方向旋转。
- 控制人物移动速度和旋转速度。
你可以根据自己的需要对这个代码进行修改,比如新增跳跃、奔跑等功能。
相关推荐







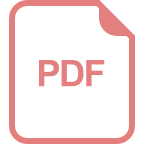
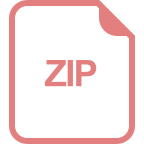
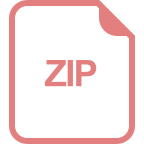
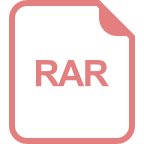