matlab对音频信号进行采样并还原,同时显示出采样前和采样后的波形
时间: 2023-06-18 17:06:17 浏览: 47
这里给出一个简单的示例代码,可以对音频信号进行采样并还原,并显示出采样前和采样后的波形。假设音频文件名为“audio.wav”,采样率为 44100 Hz,采样位数为 16 位。
```
% 读取音频文件
[x, fs] = audioread('audio.wav');
% 设定采样率和采样位数
fs_new = 8000; % 新的采样率
nbits_new = 16; % 新的采样位数
% 对音频信号进行采样
x_new = resample(x, fs_new, fs);
% 还原采样后的信号
x_recon = resample(x_new, fs, fs_new);
% 显示采样前和采样后的波形
t = (0:length(x)-1) / fs;
t_new = (0:length(x_new)-1) / fs_new;
t_recon = (0:length(x_recon)-1) / fs;
subplot(3,1,1); plot(t, x); title('Original Signal');
subplot(3,1,2); plot(t_new, x_new); title('Sampled Signal');
subplot(3,1,3); plot(t_recon, x_recon); title('Reconstructed Signal');
```
执行后,会在窗口中显示出采样前、采样后和还原后的波形图。
相关问题
matlab对音频信号进行采样并还原,显示出采样前和采样后的波形
对音频信号进行采样并还原的过程可以分为以下几步:
1.读取音频文件并绘制原始波形
```
% 读取音频文件
[y, Fs] = audioread('audio.wav');
% 绘制原始波形
t = (0:length(y)-1)/Fs; % 时间轴
figure;
plot(t, y);
xlabel('Time (s)');
ylabel('Amplitude');
title('Original Waveform');
```
2.对音频信号进行采样
```
% 设置采样率
Fs_new = 8000;
% 对音频信号进行采样
y_sampled = downsample(y, Fs/Fs_new);
% 重新计算时间轴
t_new = (0:length(y_sampled)-1)/Fs_new;
% 绘制采样后的波形
figure;
plot(t_new, y_sampled);
xlabel('Time (s)');
ylabel('Amplitude');
title('Sampled Waveform');
```
3.对采样后的信号进行还原
```
% 对采样后的信号进行插值
y_reconstructed = resample(y_sampled, Fs, Fs_new);
% 绘制还原后的波形
figure;
plot(t, y, t, y_reconstructed);
xlabel('Time (s)');
ylabel('Amplitude');
title('Reconstructed Waveform');
legend('Original', 'Reconstructed');
```
完整的代码如下:
```
% 读取音频文件
[y, Fs] = audioread('audio.wav');
% 绘制原始波形
t = (0:length(y)-1)/Fs; % 时间轴
figure;
plot(t, y);
xlabel('Time (s)');
ylabel('Amplitude');
title('Original Waveform');
% 设置采样率
Fs_new = 8000;
% 对音频信号进行采样
y_sampled = downsample(y, Fs/Fs_new);
% 重新计算时间轴
t_new = (0:length(y_sampled)-1)/Fs_new;
% 绘制采样后的波形
figure;
plot(t_new, y_sampled);
xlabel('Time (s)');
ylabel('Amplitude');
title('Sampled Waveform');
% 对采样后的信号进行插值
y_reconstructed = resample(y_sampled, Fs, Fs_new);
% 绘制还原后的波形
figure;
plot(t, y, t, y_reconstructed);
xlabel('Time (s)');
ylabel('Amplitude');
title('Reconstructed Waveform');
legend('Original', 'Reconstructed');
```
MATLAB对音频信号进行采样并还原,同时显示出采样前后的波形,保存并播放生成的音乐
以下是MATLAB代码,用于对音频信号进行采样并还原,同时显示出采样前后的波形,保存并播放生成的音乐:
```matlab
% 读取音频文件
[x, Fs] = audioread('music.wav');
% 播放原始音频
sound(x, Fs);
% 绘制原始音频波形
figure
subplot(2,1,1)
plot(x);
title('原始音频波形');
% 采样率
Fs_new = 8000;
% 采样后的音频
x_new = resample(x, Fs_new, Fs);
% 绘制采样后音频波形
subplot(2,1,2)
plot(x_new);
title('采样后音频波形');
% 保存采样后的音频
audiowrite('music_new.wav', x_new, Fs_new);
% 播放采样后的音频
sound(x_new, Fs_new);
% 还原采样后的音频
x_recovered = resample(x_new, Fs, Fs_new);
% 绘制还原后音频波形
figure
subplot(2,1,1)
plot(x);
title('原始音频波形');
subplot(2,1,2)
plot(x_recovered);
title('还原后音频波形');
% 保存还原后的音频
audiowrite('music_recovered.wav', x_recovered, Fs);
% 播放还原后的音频
sound(x_recovered, Fs);
```
需要注意的是,此代码需要将音频文件放到MATLAB当前工作目录下。如果需要修改文件路径,需要在`audioread`和`audiowrite`函数中修改路径参数。
相关推荐
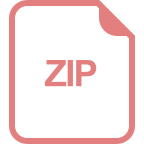
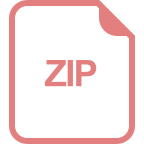












