unity 背包系统 代码
时间: 2023-05-15 18:00:26 浏览: 163
Unity背包系统的代码是一个相对复杂的程序,在实现过程中,需要先设计好背包的基本结构,包括背包中物品的数量、位置、种类等信息。其中,可以将物品抽象为一个结构体,包含物品的名称、类型、描述、图片等属性。
在具体实现时,需要以下几步操作:
1. 创建Unity场景,并在场景中添加可交互的背包界面。
2. 创建物品预制体,并将其拖拽至背包界面中,同时设置好物品的属性。
3. 定义背包的逻辑结构,包括背包容量、物品位置和数量等。
4. 编写代码实现物品的添加、删除以及响应鼠标事件进行物品的拖拽、放置等操作。
5. 实现物品之间的交换、合并、拆分等功能。
6. 加入背包数据的存储和读取功能,以实现游戏中断点保存、物品信息备份等需求。
在实现以上功能时,需要注意代码的结构清晰、可读性强,保证程序的可维护性和扩展性。同时,应加入异常处理以应对用户错误的操作和异常情况。
总之,Unity背包系统的代码实现需要具备一定的编程能力和经验,并且在实现过程中应注重程序设计和优化,以提高程序的效率和稳定性。
相关问题
unity 背包系统脚本代码
以下是一个简单的Unity背包系统脚本代码示例:
```c#
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Inventory : MonoBehaviour {
#region Singleton
public static Inventory instance;
void Awake ()
{
if (instance != null)
{
Debug.LogWarning("More than one instance of Inventory found!");
return;
}
instance = this;
}
#endregion
public delegate void OnItemChanged();
public OnItemChanged onItemChangedCallback;
public int space = 20; // 背包空间大小
public List<Item> items = new List<Item>(); // 背包物品列表
// 添加物品到背包
public bool Add (Item item)
{
if (!item.isDefaultItem) // 如果不是默认物品
{
if (items.Count >= space) // 如果背包已满
{
Debug.Log("Not enough room.");
return false;
}
items.Add(item); // 添加物品
if (onItemChangedCallback != null) // 触发背包物品改变事件
onItemChangedCallback.Invoke();
}
return true;
}
// 移除物品从背包
public void Remove (Item item)
{
items.Remove(item); // 移除物品
if (onItemChangedCallback != null) // 触发背包物品改变事件
onItemChangedCallback.Invoke();
}
}
```
该脚本包括一个Inventory类,其中包含添加物品、移除物品、背包空间大小和背包物品列表等方法和属性。使用该脚本可以轻松地创建一个简单的背包系统。
unity 背包系统
Unity背包系统是一个游戏中常用的组件,用于管理玩家在游戏中所获得的道具。一个完整的背包系统通常由数据、逻辑和UI三部分组成。在设计过程中,我们首先进行UI设计,包括背包的标题、关闭键和背包内的格子容器。
背包系统的数据部分主要负责管理物品的信息,例如物品的名称、图片、数量等。逻辑部分负责实现将物品放置进背包、对背包内物品进行管理以及使用背包内物品等功能。实际上,当我们获取或移动物品时,我们会直接修改背包数据库中的物品信息。修改完成后,我们通过背包中的UI元素来获取背包数据库中相应索引的物品信息,并将物品栏中的UI信息更新为数据库中对应序号的物品的图片和数量。
通过将背包系统分解为数据、逻辑和UI三部分,我们可以有效地管理背包系统的复杂逻辑关系,并使代码更加清晰和易于维护。这种设计模式有助于提高游戏开发的效率和代码质量。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [Unity游戏开发:背包系统的实现](https://blog.csdn.net/float_freedom/article/details/126243888)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [Unity3D RPG实现 2 —— 背包系统](https://blog.csdn.net/weixin_43757333/article/details/123187025)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
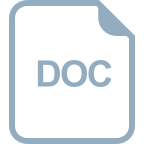
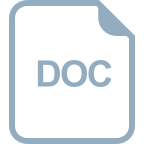
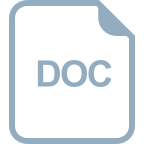
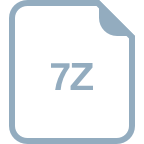
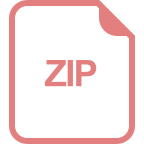
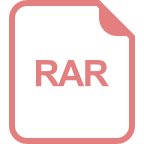
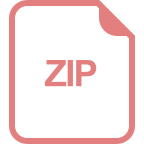
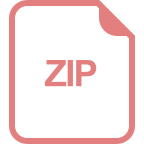
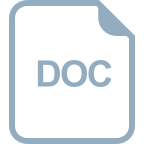
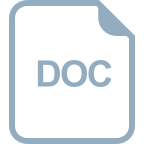




