帮我用unity写一个完善的背包系统代码
时间: 2024-05-12 14:13:06 浏览: 112
好的,我可以为您提供一个基本的背包系统代码,您可以在此基础上进行修改和完善。请注意,以下示例代码仅用于参考,实际使用时需要根据您的需求进行相应调整。
首先,我们需要一个物品类 Item:
```csharp
public class Item
{
public int id; // 物品 ID
public string name; // 物品名称
public int count; // 物品数量
public Item(int id, string name, int count)
{
this.id = id;
this.name = name;
this.count = count;
}
}
```
接下来,我们需要一个背包类 Bag:
```csharp
public class Bag
{
public List<Item> items = new List<Item>(); // 背包物品列表
public int capacity; // 背包容量
public Bag(int capacity)
{
this.capacity = capacity;
}
// 向背包中添加物品
public void AddItem(Item item)
{
if (items.Count >= capacity)
{
Debug.Log("背包已满,无法添加物品!");
return;
}
// 查找背包中是否已经存在该物品
for (int i = 0; i < items.Count; i++)
{
if (items[i].id == item.id)
{
items[i].count += item.count;
return;
}
}
// 背包中不存在该物品,直接添加
items.Add(item);
}
// 从背包中移除物品
public void RemoveItem(Item item)
{
items.Remove(item);
}
}
```
现在,我们已经有了一个基本的背包系统。您可以在 Unity 中创建一个空物体,将上述代码复制到一个新建的 C# 脚本中,然后将该脚本附加到该空物体上。接下来,您可以在 Unity 编辑器中调整该脚本的属性,例如背包容量等。
在游戏中,您可以通过以下方式来测试背包系统:
```csharp
// 创建一个背包,并设置容量为 20
Bag bag = new Bag(20);
// 创建两个物品,并添加到背包中
Item item1 = new Item(1, "金币", 10);
Item item2 = new Item(2, "药水", 5);
bag.AddItem(item1);
bag.AddItem(item2);
// 从背包中移除一个物品
bag.RemoveItem(item2);
```
以上代码仅供参考,您可以根据自己的需求进行相应的修改和扩展。如果您有任何问题或需要进一步的帮助,请随时提出。
阅读全文
相关推荐

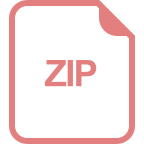
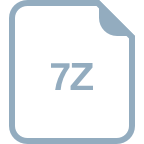
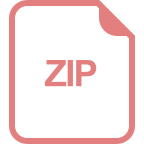
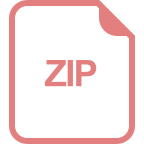
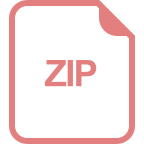
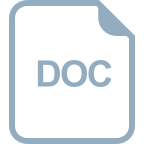







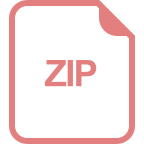
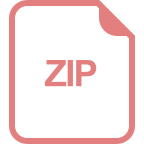