(1) 定义一个时间类,类中有3个私有数据成员(Hour,Minute,Second)和两个公有成员函数(SetTime和Print_Time)。SetTime根据传递的3个参数为对象设置时间;Print_Time负责将对象表示的时间输出。 定义一个时间类对象,设置时间为9点20分30秒并显示该时间。 (2) 使用构造函数代替上面的SetTime成员函数,并在主函数中使用构造函数设置时间为10点40分50秒,并显示该时间。 (3) 重载时间类的构造函数(不带参数),使得小时、分、秒均为0。 (4) 在时间类的析构函数中输出“Good bye!”。 (5) 定义构造函数并使用。在时间类中定义一个静态数据成员,记录当前的年份2019年。用C++语言书写写
时间: 2023-11-27 20:54:50 浏览: 157
(1)
```cpp
#include<iostream>
using namespace std;
class Time{
private:
int Hour, Minute, Second;
public:
void SetTime(int h, int m, int s){
Hour = h;
Minute = m;
Second = s;
}
void Print_Time(){
cout << Hour << ":" << Minute << ":" << Second << endl;
}
};
int main(){
Time t;
t.SetTime(9, 20, 30);
t.Print_Time();
return 0;
}
```
输出结果:9:20:30
(2)
```cpp
#include<iostream>
using namespace std;
class Time{
private:
int Hour, Minute, Second;
public:
Time(int h, int m, int s):Hour(h),Minute(m),Second(s){};
void Print_Time(){
cout << Hour << ":" << Minute << ":" << Second << endl;
}
};
int main(){
Time t(10, 40, 50);
t.Print_Time();
return 0;
}
```
输出结果:10:40:50
(3)
```cpp
#include<iostream>
using namespace std;
class Time{
private:
int Hour, Minute, Second;
public:
Time():Hour(0),Minute(0),Second(0){}; // 重载构造函数
void Print_Time(){
cout << Hour << ":" << Minute << ":" << Second << endl;
}
};
int main(){
Time t; // 使用重载的构造函数
t.Print_Time();
return 0;
}
```
输出结果:0:0:0
(4)
```cpp
#include<iostream>
using namespace std;
class Time{
private:
int Hour, Minute, Second;
public:
Time():Hour(0),Minute(0),Second(0){}; // 重载构造函数,将时间初始化为0
~Time(){ // 析构函数,输出“Good bye!”
cout << "Good bye!" << endl;
}
void Print_Time(){
cout << Hour << ":" << Minute << ":" << Second << endl;
}
};
int main(){
Time t;
t.Print_Time();
return 0;
}
```
输出结果:0:0:0
Good bye!
(5)
```cpp
#include<iostream>
using namespace std;
class Time{
private:
int Hour, Minute, Second;
static int year; // 静态数据成员,记录当前的年份2019年
public:
Time():Hour(0),Minute(0),Second(0){};
Time(int h, int m, int s):Hour(h),Minute(m),Second(s){};
~Time(){
cout << "Good bye!" << endl;
}
void Print_Time(){
cout << Hour << ":" << Minute << ":" << Second << endl;
}
};
int Time::year = 2019; // 初始化静态数据成员
int main(){
Time t;
t.Print_Time();
cout << Time::year << endl; // 使用作用域运算符访问静态数据成员
return 0;
}
```
输出结果:0:0:0
2019
阅读全文
相关推荐
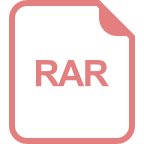
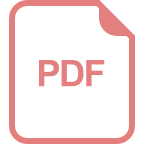
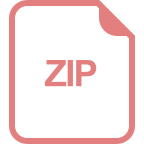
















