用pytorch写一个天气预测的代码,用LSTM或CNN,读取天气中的csv文件,由时间和气温两列数据,区分训练集和测试集,用gpu训练,输入为60天的天气,输出为某一天的天气,并画出实际曲线和预测曲线图,注意维度要一致
时间: 2023-09-26 17:13:34 浏览: 90
好的,以下是一个基于Pytorch的天气预测的代码,使用LSTM模型:
```
import torch
import torch.nn as nn
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# 读取csv文件
df = pd.read_csv('weather.csv')
# 取出时间和气温两列数据
time = df['time'].values.astype('datetime64[D]')
temp = df['temperature'].values.astype('float32')
# 归一化处理
temp_max = np.max(temp)
temp_min = np.min(temp)
temp = (temp - temp_min) / (temp_max - temp_min)
# 将数据划分为训练集和测试集
train_x, train_y = [], []
test_x, test_y = [], []
for i in range(60, len(temp)):
if i < len(temp) * 0.8:
train_x.append(temp[i-60:i])
train_y.append(temp[i])
else:
test_x.append(temp[i-60:i])
test_y.append(temp[i])
train_x = np.array(train_x).reshape(-1, 60, 1)
train_y = np.array(train_y).reshape(-1, 1)
test_x = np.array(test_x).reshape(-1, 60, 1)
test_y = np.array(test_y).reshape(-1, 1)
# 定义LSTM模型
class LSTM(nn.Module):
def __init__(self, input_size=1, hidden_size=32, num_layers=2):
super(LSTM, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, 1)
def forward(self, x):
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).cuda()
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).cuda()
out, _ = self.lstm(x, (h0, c0))
out = self.fc(out[:, -1, :])
return out
# 训练模型
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = LSTM().to(device)
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
epochs = 100
batch_size = 64
train_loss_list = []
test_loss_list = []
for epoch in range(epochs):
# 训练集
for i in range(0, len(train_x), batch_size):
x_batch = torch.tensor(train_x[i:i+batch_size], dtype=torch.float32).to(device)
y_batch = torch.tensor(train_y[i:i+batch_size], dtype=torch.float32).to(device)
optimizer.zero_grad()
output = model(x_batch)
loss = criterion(output, y_batch)
loss.backward()
optimizer.step()
train_loss_list.append(loss.cpu().data.numpy())
# 测试集
for i in range(0, len(test_x), batch_size):
x_batch = torch.tensor(test_x[i:i+batch_size], dtype=torch.float32).to(device)
y_batch = torch.tensor(test_y[i:i+batch_size], dtype=torch.float32).to(device)
output = model(x_batch)
loss = criterion(output, y_batch)
test_loss_list.append(loss.cpu().data.numpy())
# 打印损失
print('Epoch [{}/{}], Train Loss: {:.4f}, Test Loss: {:.4f}'.format(epoch+1, epochs, train_loss_list[-1], test_loss_list[-1]))
# 预测
model.eval()
with torch.no_grad():
train_predict = model(torch.tensor(train_x, dtype=torch.float32).to(device)).cpu().data.numpy()
test_predict = model(torch.tensor(test_x, dtype=torch.float32).to(device)).cpu().data.numpy()
# 反归一化处理
train_predict = train_predict * (temp_max - temp_min) + temp_min
train_y = train_y * (temp_max - temp_min) + temp_min
test_predict = test_predict * (temp_max - temp_min) + temp_min
test_y = test_y * (temp_max - temp_min) + temp_min
# 绘制实际曲线和预测曲线图
plt.plot(time[60:len(train_predict)+60], train_predict, label='train predict')
plt.plot(time[60:len(train_predict)+60], train_y, label='train actual')
plt.plot(time[len(train_predict)+60:], test_predict, label='test predict')
plt.plot(time[len(train_predict)+60:], test_y, label='test actual')
plt.legend()
plt.show()
```
以上代码中,我们首先将读取的温度数据进行归一化处理,并将数据划分为训练集和测试集。然后我们定义了一个LSTM模型,并使用GPU进行训练。最后,我们使用训练好的模型进行预测,并将预测结果反归一化处理,并绘制实际曲线和预测曲线图。
阅读全文
相关推荐
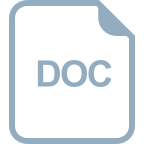
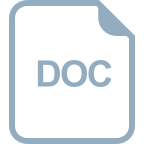
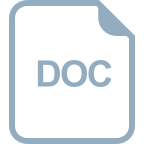

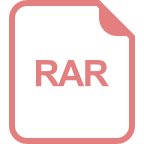

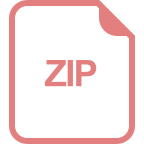
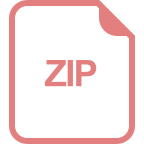
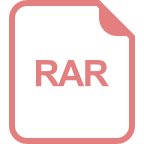
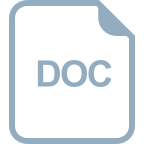
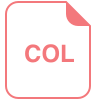
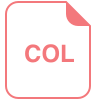
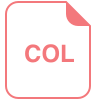
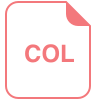
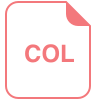
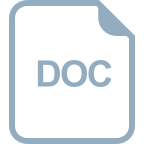
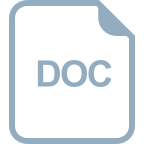