使用Python的json模块来解析JSON文件详细代码
时间: 2023-09-06 14:04:59 浏览: 162
### 回答1:
可以使用下面的代码来解析JSON文件:import jsonwith open('example.json', 'r') as f:
data = json.load(f)# 访问数据
for key, value in data.items():
print('{0} : {1}'.format(key, value))
### 回答2:
使用Python中的json模块来解析JSON文件需要以下步骤:
1. 导入json模块:
```python
import json
```
2. 打开JSON文件:
```python
with open('example.json') as file:
data = json.load(file)
```
这里假设JSON文件名为example.json,并使用open函数打开文件。
3. 解析JSON数据:
现在可以使用Python索引和键值对的方式来访问JSON数据。例如,假设JSON文件包含一个名为"person"的顶级键,其中包含一个名为"name"的嵌套键:
```python
name = data['person']['name']
```
这将返回"person"键下的"name"键对应的值。
4. 循环遍历JSON数据:
如果JSON文件包含一个名为"items"的列表,我们可以使用for循环来遍历并获取每个条目的值:
```python
items = data['items']
for item in items:
print(item['name'])
```
这将打印出列表中每个元素的"name"键对应的值。
5. 将数据写入JSON文件:
如果需要将数据写入JSON文件,可以使用json.dump函数:
```python
data = {'name': 'John', 'age': 30}
with open('output.json', 'w') as file:
json.dump(data, file)
```
这将将字典对象"data"写入名为"output.json"的文件中。
以上是使用Python的json模块来解析JSON文件的详细代码。请根据实际情况修改文件名、键名、变量名等。
### 回答3:
使用Python的json模块来解析JSON文件的详细代码如下:
```python
import json
# 读取JSON文件
with open('data.json', 'r') as file:
json_data = json.load(file)
# 解析JSON数据
for data in json_data:
name = data['name']
age = data['age']
city = data['city']
print("Name: ", name)
print("Age: ", age)
print("City: ", city)
print("")
# 更新JSON数据
json_data[0]['age'] = 25
# 写入更新后的JSON到文件
with open('data_updated.json', 'w') as file:
json.dump(json_data, file)
print("Updated JSON data written to file.")
```
以上代码首先使用`json.load()`函数读取名为"data.json"的JSON文件并保存到变量`json_data`中。
然后,我们可以使用循环遍历`json_data`中的每个数据项,并使用键来访问对应的值。在这个例子中,我们假设JSON数据的格式如下:
```json
[
{
"name": "John Doe",
"age": 30,
"city": "New York"
},
{
"name": "Jane Smith",
"age": 25,
"city": "Chicago"
}
]
```
在循环中,我们使用`data['name']`、`data['age']`和`data['city']`来访问每个数据项的姓名、年龄和城市,并将其打印输出。
接下来,我们对JSON数据进行了更新,将第一个数据项的年龄改为25。
最后,我们使用`json.dump()`函数将更新后的JSON数据写入一个名为"data_updated.json"的新文件中。
阅读全文
相关推荐
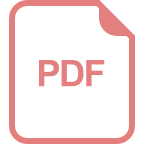
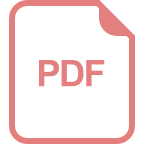
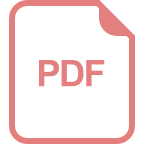













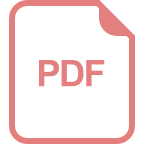
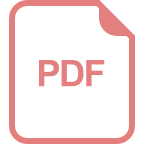
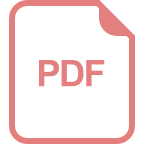