python写一个背单词软件
时间: 2024-11-30 15:20:34 浏览: 23
在Python中编写一个简单的背单词软件,可以利用字典数据结构存储单词列表,并结合随机选择、提示、复习等功能。这里提供一个基础框架的示例:
```python
import random
class Flashcard:
def __init__(self, word, definition):
self.word = word
self.definition = definition
self.revealed = False
class VocabularyApp:
def __init__(self):
self.words = ['apple', 'banana', 'cherry', ...] # 这里可以替换为实际的单词列表
self.current_card_index = 0
def shuffle_words(self):
random.shuffle(self.words)
def show_card(self):
if not self.current_card_index < len(self.words):
return "No more cards left!"
card = self.words[self.current_card_index]
if not card.revealed:
return f"Word: {card.word}\nDefinition: (hide for review)"
else:
return f"You have already reviewed '{card.word}'.\nNext card?"
def reveal_definition(self):
card = self.words[self.current_card_index]
card.revealed = True
return f"The definition is: {card.definition}"
def review(self):
card = self.show_card()
if "Next card?" in card:
self.current_card_index += 1
else:
return self.reveal_definition()
# 使用示例
app = VocabularyApp()
app.shuffle_words()
while True:
print(app.show_card())
user_input = input("Enter 'r' to review or any other key to continue: ")
if user_input.lower() == 'r':
app.review()
```
这个示例只是一个基本的框架,你可以添加用户输入验证、计分系统、学习进度跟踪等更高级的功能。
阅读全文
相关推荐
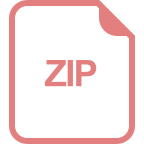
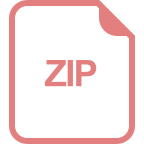


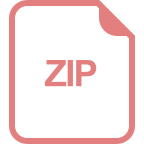
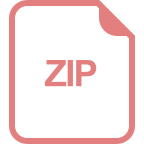
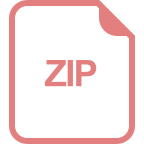
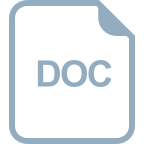
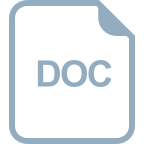
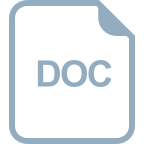
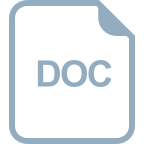





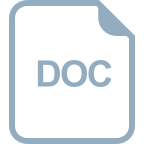
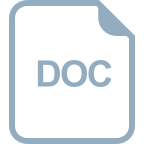