H5获取安卓原生蓝牙监听数据
时间: 2023-07-29 16:14:33 浏览: 131
在安卓应用中,可以通过以下步骤获取原生蓝牙监听数据:
1. 首先,需要在 AndroidManifest.xml 文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
```
2. 然后,在应用中获取 BluetoothAdapter 对象,并启动蓝牙:
```java
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (bluetoothAdapter == null) {
// 设备不支持蓝牙
return;
}
if (!bluetoothAdapter.isEnabled()) {
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
```
3. 接下来,需要实现 BluetoothGattCallback 类来监听蓝牙设备的连接状态以及数据传输:
```java
private BluetoothGattCallback mBluetoothGattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
super.onConnectionStateChange(gatt, status, newState);
// 蓝牙设备连接状态发生改变时的回调函数
if (newState == BluetoothProfile.STATE_CONNECTED) {
// 设备已连接
gatt.discoverServices();
} else if (newState == BluetoothProfile.STATE_DISCONNECTED) {
// 设备已断开连接
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
super.onServicesDiscovered(gatt, status);
// 发现服务时的回调函数
if (status == BluetoothGatt.GATT_SUCCESS) {
List<BluetoothGattService> services = gatt.getServices();
for (BluetoothGattService service : services) {
List<BluetoothGattCharacteristic> characteristics = service.getCharacteristics();
for (BluetoothGattCharacteristic characteristic : characteristics) {
// 订阅特定的特征值,以接收数据
if (characteristic.getUuid().equals(UUID.fromString(CHARACTERISTIC_UUID))) {
gatt.setCharacteristicNotification(characteristic, true);
BluetoothGattDescriptor descriptor = characteristic.getDescriptor(UUID.fromString(CLIENT_CHARACTERISTIC_CONFIG));
descriptor.setValue(BluetoothGattDescriptor.ENABLE_NOTIFICATION_VALUE);
gatt.writeDescriptor(descriptor);
}
}
}
}
}
@Override
public void onCharacteristicChanged(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic) {
super.onCharacteristicChanged(gatt, characteristic);
// 接收到数据时的回调函数
byte[] data = characteristic.getValue();
// 处理数据
}
};
```
4. 最后,在应用中连接蓝牙设备并开始监听数据:
```java
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(deviceAddress);
BluetoothGatt gatt = device.connectGatt(this, false, mBluetoothGattCallback);
```
阅读全文
相关推荐
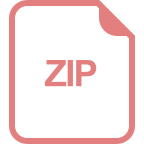
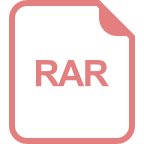
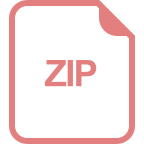















