H5获取Android蓝牙监听
时间: 2023-07-30 18:04:14 浏览: 154
要在H5中获取Android蓝牙监听,需要通过JavaScript与原生Android代码进行交互。具体步骤如下:
1. 在Android端实现蓝牙监听功能,并创建一个JavaScript接口,在接口中将蓝牙监听数据传递给H5页面。
2. 在H5页面中使用JavaScript调用Android端的接口,获取蓝牙监听数据并处理。
以下是一个简单的示例代码:
在Android端实现蓝牙监听功能:
```java
public class BluetoothListener {
private static BluetoothListener instance;
private BluetoothAdapter bluetoothAdapter;
private BluetoothDevice bluetoothDevice;
private BluetoothSocket bluetoothSocket;
private InputStream inputStream;
private OutputStream outputStream;
private boolean isListening = false;
public static BluetoothListener getInstance() {
if (instance == null) {
instance = new BluetoothListener();
}
return instance;
}
public void startListening() {
try {
bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
bluetoothDevice = bluetoothAdapter.getRemoteDevice(DEVICE_ADDRESS);
bluetoothSocket = bluetoothDevice.createRfcommSocketToServiceRecord(UUID.fromString(SERVICE_UUID));
bluetoothSocket.connect();
inputStream = bluetoothSocket.getInputStream();
outputStream = bluetoothSocket.getOutputStream();
isListening = true;
while (isListening) {
byte[] buffer = new byte[1024];
int count = inputStream.read(buffer);
if (count > 0) {
String data = new String(buffer, 0, count);
sendBluetoothDataToJS(data);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
private void sendBluetoothDataToJS(String data) {
// 将蓝牙监听数据传递给H5页面
String js = "javascript:handleBluetoothData('" + data + "')";
MainActivity.getInstance().runOnUiThread(() -> {
MainActivity.getInstance().getWebView().loadUrl(js);
});
}
public void stopListening() {
isListening = false;
try {
if (inputStream != null) {
inputStream.close();
}
if (outputStream != null) {
outputStream.close();
}
if (bluetoothSocket != null) {
bluetoothSocket.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在H5页面中使用JavaScript调用Android端的接口,获取蓝牙监听数据并处理:
```javascript
function handleBluetoothData(data) {
// 处理蓝牙监听数据
console.log(data);
}
function startBluetoothListening() {
// 调用Android端的接口开始监听蓝牙数据
Android.startListening();
}
function stopBluetoothListening() {
// 调用Android端的接口停止监听蓝牙数据
Android.stopListening();
}
```
需要注意的是,在Android端实现蓝牙监听功能时需要获取蓝牙相关的权限,例如:
```xml
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
```
阅读全文
相关推荐
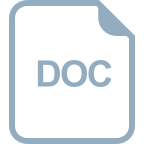
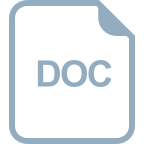
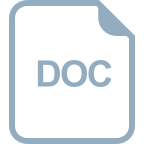

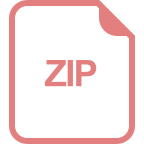
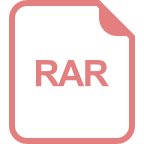
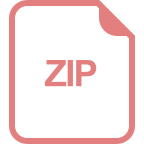
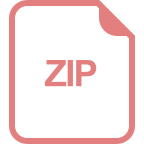
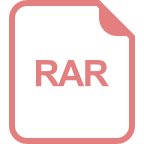
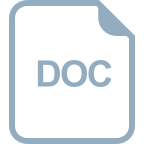
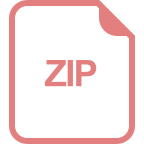
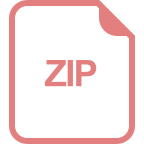
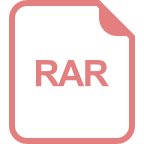
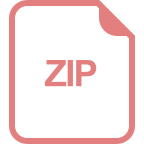
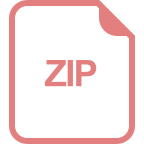
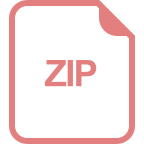
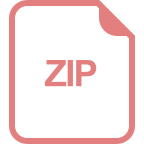
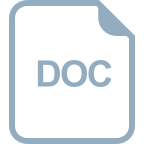
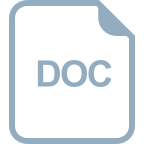