python图像识别带有gui
时间: 2024-12-25 14:19:06 浏览: 3
### Python 图像识别 GUI 实现方法
#### 使用PyQt构建图形用户界面
为了创建一个功能齐全的图像识别应用程序,通常会选择使用成熟的GUI框架。PyQt是一个强大的跨平台Python库,用于开发桌面应用程序。它提供了丰富的组件集和信号槽机制,使得开发者可以轻松地设计复杂的用户交互界面[^4]。
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton, QVBoxLayout, QWidget
from PyQt5.QtGui import QPixmap
class ImageRecognitionApp(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Image Recognition Tool')
layout = QVBoxLayout()
# Add widgets to the layout here...
label = QLabel(self)
pixmap = QPixmap('path_to_image.jpg') # Replace with your image path.
label.setPixmap(pixmap)
button = QPushButton('Recognize', self)
button.clicked.connect(self.on_click)
widget = QWidget()
widget.setLayout(layout)
self.setCentralWidget(widget)
def on_click():
print("Button clicked")
if __name__ == '__main__':
app = QApplication(sys.argv)
window = ImageRecognitionApp()
window.show()
sys.exit(app.exec_())
```
这段代码展示了如何初始化一个基本的应用程序窗口并设置其标题为“Image Recognition Tool”。还定义了一个按钮点击事件处理器`on_click()`函数,当用户按下“Recognize”按钮时会触发该函数执行特定操作。
#### 集成OpenCV进行图像处理与分析
对于图像识别部分,则依赖于计算机视觉领域广泛使用的开源库—OpenCV (Open Source Computer Vision Library) 。此库不仅支持多种编程语言接口,而且拥有大量预置算法可用于特征提取、对象检测等任务。下面是一些基础的例子说明怎样加载图片以及显示出来:
```python
import cv2
img = cv2.imread('example.png', 0) # Read as grayscale
cv2.imshow('Example Window Title', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
上述脚本读取了一张名为'example.png' 的灰度图,并在一个新弹出的小窗内展示给用户查看。需要注意的是,在实际部署过程中应当考虑更优雅的方式关闭这些临时视窗而不是简单调用destroyAllWindows() 方法[^2]。
#### 结合深度学习模型提升准确性
如果目标不仅仅是简单的模式匹配而是更加复杂的情景比如分类或者定位物体的位置,那么引入深度神经网络将会极大地提高系统的性能表现。以卷积神经网络(CNNs)为例,它们特别擅长处理二维结构化数据如照片视频帧之类的东西。MNIST手写数字识别就是一个很好的入门案例[^1]。
```python
import tensorflow as tf
from keras.models import Sequential
from keras.layers import Dense, Conv2D, Flatten, MaxPooling2D
model = Sequential([
Conv2D(filters=32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(units=128, activation='relu'),
Dense(units=10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Assuming X_train and y_train are preprocessed datasets from MNIST dataset
history = model.fit(X_train, y_train, epochs=5)
```
这里给出了一份简化版的手写字体辨识器搭建指南,其中包含了建立多层感知机架构的过程及其参数调整建议。当然这只是一个起点;随着经验积累和技术进步,还可以探索更多高级特性来优化最终成果。
阅读全文
相关推荐
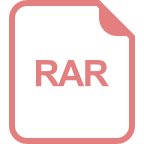
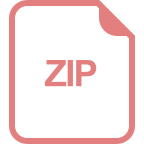
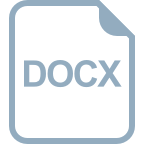
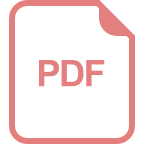
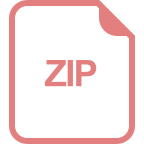
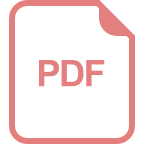
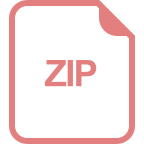
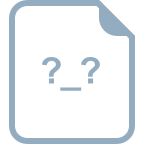
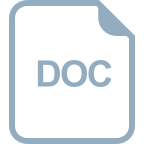
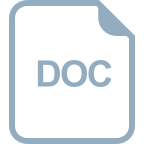
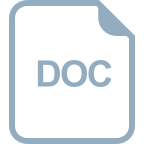
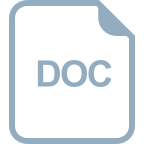
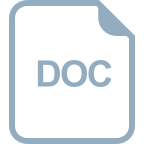
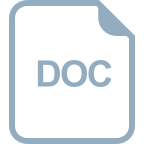
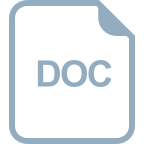
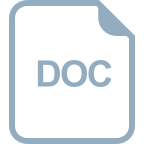
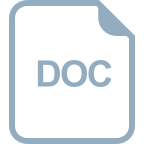
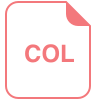
