单链表的查找与插入代码c语言
时间: 2023-12-24 17:02:40 浏览: 20
单链表的查找与插入代码C语言实现如下:
1. 定义单链表节点结构体
```c
struct ListNode {
int val;
struct ListNode *next;
};
```
2. 查找指定值的节点
```c
struct ListNode* searchList(struct ListNode *head, int target) {
struct ListNode *cur = head;
while (cur != NULL && cur->val != target) {
cur = cur->next;
}
return cur;
}
```
3. 在指定位置插入节点
```c
void insertNode(struct ListNode **head, int pos, int val) {
struct ListNode *newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->val = val;
newNode->next = NULL;
if (pos == 0) {
newNode->next = *head;
*head = newNode;
return;
}
struct ListNode *prev = NULL;
struct ListNode *cur = *head;
int i = 0;
while (cur != NULL && i < pos) {
prev = cur;
cur = cur->next;
i++;
}
prev->next = newNode;
newNode->next = cur;
}
```
完整代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* searchList(struct ListNode *head, int target) {
struct ListNode *cur = head;
while (cur != NULL && cur->val != target) {
cur = cur->next;
}
return cur;
}
void insertNode(struct ListNode **head, int pos, int val) {
struct ListNode *newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->val = val;
newNode->next = NULL;
if (pos == 0) {
newNode->next = *head;
*head = newNode;
return;
}
struct ListNode *prev = NULL;
struct ListNode *cur = *head;
int i = 0;
while (cur != NULL && i < pos) {
prev = cur;
cur = cur->next;
i++;
}
prev->next = newNode;
newNode->next = cur;
}
void printList(struct ListNode *head) {
struct ListNode *cur = head;
while (cur != NULL) {
printf("%d ", cur->val);
cur = cur->next;
}
printf("\n");
}
int main() {
struct ListNode *head = NULL;
insertNode(&head, 0, 1);
insertNode(&head, 1, 2);
insertNode(&head, 2, 3);
printList(head);
struct ListNode *target = searchList(head, 2);
if (target != NULL) {
printf("Found target node with value %d\n", target->val);
} else {
printf("Target node not found\n");
}
return 0;
}
```
相关推荐
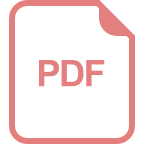
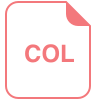
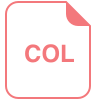
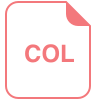
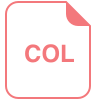
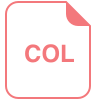









