单链表创建、删除、查找、插入之C语言实现
时间: 2023-07-10 19:14:25 浏览: 103
单链表是一种链式存储结构,由一个个结点构成,每个结点包含两个域:数据域和指针域。数据域用于存储数据,指针域用于指向下一个结点。以下是单链表的基本操作的C语言实现:
1.创建链表
```c
typedef struct node {
int data;
struct node *next;
} Node;
Node *createList() {
Node *head = NULL;
Node *tail = NULL;
int num;
printf("请输入链表的数据,以-1结束:");
scanf("%d", &num);
while (num != -1) {
Node *p = (Node*)malloc(sizeof(Node));
p->data = num;
p->next = NULL;
if (head == NULL) {
head = p;
tail = p;
} else {
tail->next = p;
tail = p;
}
scanf("%d", &num);
}
return head;
}
```
2.删除结点
```c
Node *deleteNode(Node *head, int num) {
Node *p = head;
Node *prev = NULL;
while (p != NULL && p->data != num) {
prev = p;
p = p->next;
}
if (p == NULL) {
printf("链表中没有该结点\n");
return head;
}
if (prev == NULL) {
head = head->next;
} else {
prev->next = p->next;
}
free(p);
return head;
}
```
3.查找结点
```c
Node *searchNode(Node *head, int num) {
Node *p = head;
while (p != NULL && p->data != num) {
p = p->next;
}
if (p == NULL) {
printf("链表中没有该结点\n");
return NULL;
}
return p;
}
```
4.插入结点
```c
Node *insertNode(Node *head, int num, int index) {
Node *p = (Node*)malloc(sizeof(Node));
p->data = num;
p->next = NULL;
if (head == NULL) {
if (index == 0) {
return p;
} else {
printf("链表为空,不能插入结点\n");
free(p);
return NULL;
}
}
if (index == 0) {
p->next = head;
return p;
}
Node *prev = head;
Node *next = head->next;
int i = 1;
while (next != NULL && i != index) {
prev = prev->next;
next = next->next;
i++;
}
if (i != index) {
printf("插入位置超出链表长度\n");
free(p);
return head;
}
prev->next = p;
p->next = next;
return head;
}
```
相关推荐
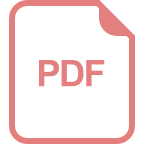
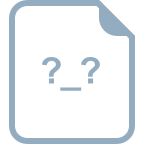














