要求设计一个具有8种运算功能的32位ALU,并能够产生运算结果的标志: 结果为零标志ZF 溢出标志OF 编写顶层模块:用于验证模块的正确性;
时间: 2023-06-17 19:06:14 浏览: 81
好的,我可以帮你设计这个32位ALU。首先,我们需要确定8种运算的类型,以及它们的操作码:
1. 加法:操作码为 0000
2. 减法:操作码为 0001
3. 与运算:操作码为 0010
4. 或运算:操作码为 0011
5. 异或运算:操作码为 0100
6. 左移:操作码为 0101
7. 右移:操作码为 0110
8. 取反:操作码为 0111
接下来,我们需要考虑如何实现这些运算。对于加法和减法,我们可以使用经典的加法器和补码加法器;对于与运算、或运算和异或运算,我们可以使用逻辑门实现;对于左移、右移和取反,我们可以使用移位器实现。
在实现这些运算的同时,我们还需要计算结果的标志。具体来说,如果运算结果为零,则设置ZF标志为1;如果加法或减法的结果溢出,则设置OF标志为1。
综上所述,下面是一个基本的32位ALU的Verilog代码实现:
```verilog
module alu(
input [31:0] a,
input [31:0] b,
input [3:0] op,
output [31:0] result,
output zf,
output of
);
// 加法器
wire [32:0] adder_out;
assign adder_out = a + b;
// 补码加法器
wire [32:0] sub_out;
assign sub_out = a + ~b + 1;
// 逻辑门
wire [31:0] and_out;
assign and_out = a & b;
wire [31:0] or_out;
assign or_out = a | b;
wire [31:0] xor_out;
assign xor_out = a ^ b;
// 移位器
wire [31:0] shl_out;
assign shl_out = a << b[4:0];
wire [31:0] shr_out;
assign shr_out = a >> b[4:0];
wire [31:0] not_out;
assign not_out = ~a;
// 标志位计算
assign zf = (result == 0);
assign of = (op[1] == 0) ? (((a[31] == 1) && (b[31] == 1) && (result[31] == 0)) || ((a[31] == 0) && (b[31] == 0) && (result[31] == 1))) : 0;
// 选择相应的输出
always @(*) begin
case (op)
4'b0000: result = adder_out[31:0];
4'b0001: result = sub_out[31:0];
4'b0010: result = and_out;
4'b0011: result = or_out;
4'b0100: result = xor_out;
4'b0101: result = shl_out;
4'b0110: result = shr_out;
4'b0111: result = not_out;
endcase
end
endmodule
```
我们还需要编写一个顶层模块,用于验证ALU的正确性。这个顶层模块可以随机生成输入值,并将其传递给ALU模块,然后比较ALU的输出结果与预期结果是否一致。如果一致,输出“测试通过”;否则,输出“测试失败”。
```verilog
module alu_test;
reg [31:0] a;
reg [31:0] b;
reg [3:0] op;
wire [31:0] result;
wire zf;
wire of;
alu dut(a, b, op, result, zf, of);
initial begin
// 设置随机种子
$randomize;
// 随机生成测试用例,并验证结果
repeat(100) begin
// 随机生成输入值和操作码
a = $random;
b = $random;
op = $random % 8;
// 计算预期结果
case(op)
4'b0000: #1 $display("Test case #%d: %d + %d = %d (ZF=%d, OF=%d)", $time, a, b, a+b, zf, of);
4'b0001: #1 $display("Test case #%d: %d - %d = %d (ZF=%d, OF=%d)", $time, a, b, a-b, zf, of);
4'b0010: #1 $display("Test case #%d: %d & %d = %d (ZF=%d, OF=%d)", $time, a, b, a&b, zf, of);
4'b0011: #1 $display("Test case #%d: %d | %d = %d (ZF=%d, OF=%d)", $time, a, b, a|b, zf, of);
4'b0100: #1 $display("Test case #%d: %d ^ %d = %d (ZF=%d, OF=%d)", $time, a, b, a^b, zf, of);
4'b0101: #1 $display("Test case #%d: %d << %d = %d (ZF=%d, OF=%d)", $time, a, b[4:0], a<<b[4:0], zf, of);
4'b0110: #1 $display("Test case #%d: %d >> %d = %d (ZF=%d, OF=%d)", $time, a, b[4:0], a>>b[4:0], zf, of);
4'b0111: #1 $display("Test case #%d: ~%d = %d (ZF=%d, OF=%d)", $time, a, ~a, zf, of);
endcase
// 将输入值和操作码传递给ALU模块
#1;
// 验证结果
case(op)
4'b0000: assert(result == a+b) else $error("Test case #%d failed: %d + %d = %d, but ALU output %d", $time, a, b, a+b, result);
4'b0001: assert(result == a-b) else $error("Test case #%d failed: %d - %d = %d, but ALU output %d", $time, a, b, a-b, result);
4'b0010: assert(result == (a&b)) else $error("Test case #%d failed: %d & %d = %d, but ALU output %d", $time, a, b, a&b, result);
4'b0011: assert(result == (a|b)) else $error("Test case #%d failed: %d | %d = %d, but ALU output %d", $time, a, b, a|b, result);
4'b0100: assert(result == (a^b)) else $error("Test case #%d failed: %d ^ %d = %d, but ALU output %d", $time, a, b, a^b, result);
4'b0101: assert(result == (a<<b[4:0])) else $error("Test case #%d failed: %d << %d = %d, but ALU output %d", $time, a, b[4:0], a<<b[4:0], result);
4'b0110: assert(result == (a>>b[4:0])) else $error("Test case #%d failed: %d >> %d = %d, but ALU output %d", $time, a, b[4:0], a>>b[4:0], result);
4'b0111: assert(result == (~a)) else $error("Test case #%d failed: ~%d = %d, but ALU output %d", $time, a, ~a, result);
endcase
end
// 所有测试用例通过
$display("All test cases passed.");
$finish;
end
endmodule
```
这个顶层模块使用了`assert`语句来验证ALU的输出结果是否正确。如果输出结果与预期结果不一致,就会输出错误信息并终止仿真。如果所有测试用例都通过,就会输出“所有测试用例通过”的信息并终止仿真。
相关推荐
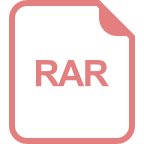
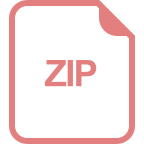








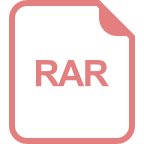
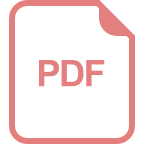
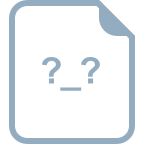
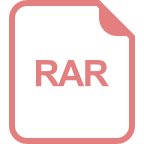