十进制转九进制c语言代码
时间: 2024-11-20 14:24:44 浏览: 9
在C语言中,将十进制数转换成九进制可以采用循环和除法的方式实现。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h>
// Function to convert decimal to octal
char* decimal_to_octal(int num) {
char result[50]; // Array to store the octal number as string
int i = 0;
if (num == 0)
return "0"; // If input is zero, return '0'
while(num > 0) {
result[i++] = (num % 8) + '0'; // Convert remainder to character and append
num /= 8; // Divide by 8 for next iteration
}
result[i] = '\0'; // Add null terminator
// Reverse the string as octal representation starts from rightmost digit
reverse(result, i);
return result;
}
// Helper function to reverse a string in-place
void reverse(char* str, int len) {
int start = 0;
int end = len - 1;
while(start < end) {
char temp = str[start];
str[start] = str[end];
str[end] = temp;
start++;
end--;
}
}
int main() {
int decimal_num;
printf("Enter a decimal number: ");
scanf("%d", &decimal_num);
char* octal_num = decimal_to_octal(decimal_num);
printf("Octal equivalent of %d is: %s\n", decimal_num, octal_num);
free(octal_num); // Don't forget to free dynamically allocated memory
return 0;
}
```
在这个程序中,`decimal_to_octal`函数负责将输入的十进制数字转换为八进制字符串,然后主函数中获取用户输入并打印结果。
阅读全文
相关推荐
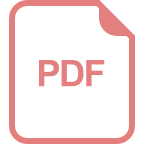
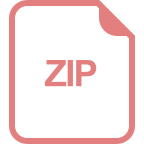
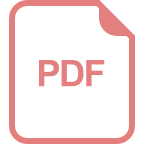
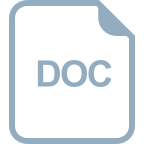














