Java实现将语音文件作为HTTP响应返回给前端
时间: 2024-11-25 17:10:06 浏览: 4
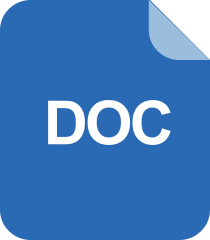
java实现聊天系统(计算机网络课程设计报告).doc
在Java中,将语音文件作为HTTP响应返回给前端,通常会用到Servlet或者Spring MVC这类Web服务框架。以下是一个基本的例子,假设使用的是Spring Boot:
1. **配置Spring MVC处理器**:
首先,在Spring Boot的`application.properties`中启用Multipart配置,以便支持文件上传:
```properties
spring.mvc.multipart.enabled=true
```
2. **创建Controller**:
创建一个新的Controller类,比如`VoiceController`,在其中添加一个处理GET请求的方法,用于提供语音文件:
```java
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.multipart.MultipartFile;
@GetMapping("/download/audio")
public ResponseEntity<byte[]> downloadAudio(@RequestParam("file") MultipartFile file) {
// 检查文件是否有效
if (file.isEmpty()) {
throw new IllegalArgumentException("File is empty");
}
// 将文件转换为字节数组
byte[] audioBytes = file.getBytes();
// 设置Content-Disposition头,告诉浏览器这是一个下载请求
HttpHeaders headers = new HttpHeaders();
headers.setContentDispositionFormData("filename", file.getOriginalFilename());
// 返回字节数组,指定Content-Type为音频文件的MIME类型
return ResponseEntity.ok()
.headers(headers)
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(audioBytes);
}
```
这里假设`file`参数是从前端传递过来的音频文件。
3. **前端接收**:
前端使用AJAX或fetch请求这个路径,并将响应保存到`<audio>`元素中播放:
```javascript
axios.get('/download/audio?file=<file-name>', {
responseType: 'arraybuffer'
}).then(response => {
const audioBlob = new Blob([response.data], { type: 'audio/*' });
const audioURL = window.URL.createObjectURL(audioBlob);
document.getElementById('audio-player').src = audioURL;
});
```
阅读全文
相关推荐
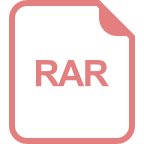
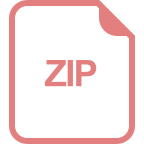

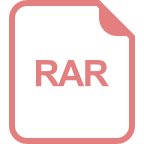
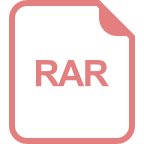
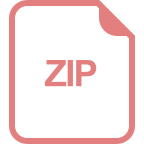
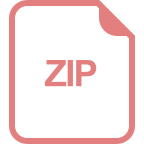
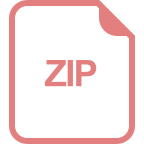
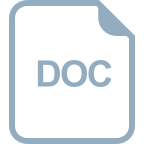
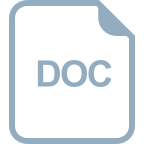
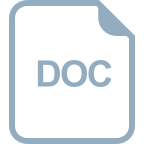
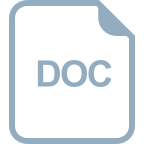
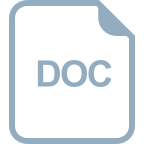
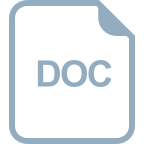
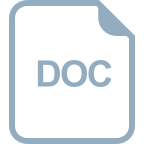
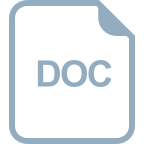
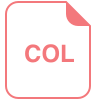
