用c#写一段识别视频中的几何形状识别
时间: 2023-06-18 17:02:41 浏览: 127
要实现视频中几何形状的识别,需要进行以下步骤:
1. 读取视频帧:使用C# 的图像处理库OpenCVSharp读取视频帧。
2. 图像预处理:对读取的视频帧进行预处理,包括灰度化、二值化、形态学处理等,以便更好地提取几何形状。
3. 轮廓检测:使用OpenCVSharp的轮廓检测功能,提取出图像中的轮廓。
4. 形状识别:对提取出的轮廓进行形状识别,可以使用OpenCVSharp的形状匹配函数进行匹配,判断轮廓是否为矩形、圆形、三角形等几何形状。
5. 标记结果:将识别结果标记在图像上,可以使用OpenCVSharp的绘图功能。
下面是一份用C#实现的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using OpenCvSharp;
namespace ShapeDetection
{
class Program
{
static void Main(string[] args)
{
// 读取视频帧
VideoCapture capture = new VideoCapture("test.avi");
Mat frame = new Mat();
// 创建窗口
Cv2.NamedWindow("Shape Detection", WindowFlags.Normal);
while (true)
{
// 读取一帧
capture.Read(frame);
if (frame.Empty())
{
break;
}
// 图像预处理
Mat gray = new Mat();
Cv2.CvtColor(frame, gray, ColorConversionCodes.BGR2GRAY);
Mat binary = new Mat();
Cv2.Threshold(gray, binary, 0, 255, ThresholdTypes.Binary | ThresholdTypes.Otsu);
Mat morphology = new Mat();
Mat kernel = Cv2.GetStructuringElement(MorphShapes.Rect, new Size(3, 3));
Cv2.MorphologyEx(binary, morphology, MorphTypes.Open, kernel);
// 轮廓检测
Point[][] contours;
HierarchyIndex[] hierarchy;
Cv2.FindContours(morphology, out contours, out hierarchy, RetrievalModes.Tree, ContourApproximationModes.ApproxSimple);
// 形状识别
for (int i = 0; i < contours.Length; i++)
{
Point[] contour = contours[i];
double perimeter = Cv2.ArcLength(contour, true);
Point[] approx = Cv2.ApproxPolyDP(contour, 0.02 * perimeter, true);
Moments moments = Cv2.Moments(contour, false);
Point2f center = new Point2f((float)(moments.M10 / moments.M00), (float)(moments.M01 / moments.M00));
if (approx.Length >= 3 && approx.Length <= 6)
{
// 判断是否为矩形
Rect rect = Cv2.BoundingRect(approx);
double aspectRatio = (double)rect.Width / rect.Height;
if (aspectRatio >= 0.95 && aspectRatio <= 1.05)
{
Cv2.Rectangle(frame, rect, Scalar.Green, 2);
}
// 判断是否为三角形
else if (approx.Length == 3)
{
Cv2.Polylines(frame, new Point[][] { approx }, true, Scalar.Red, 2);
}
}
// 判断是否为圆形
else
{
double area = Cv2.ContourArea(contour);
double radius = Math.Sqrt(area / Math.PI);
if (Math.Abs(perimeter - Math.PI * 2 * radius) < 0.1 * perimeter)
{
Cv2.Circle(frame, (Point)center, (int)radius, Scalar.Blue, 2);
}
}
}
// 显示结果
Cv2.ImShow("Shape Detection", frame);
Cv2.WaitKey(30);
}
// 释放资源
capture.Release();
Cv2.DestroyAllWindows();
}
}
}
```
该代码实现了对视频中的矩形、圆形和三角形的识别,并将识别结果标记在图像上。
阅读全文
相关推荐
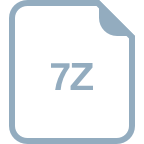

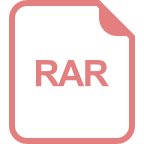
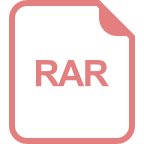
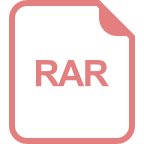
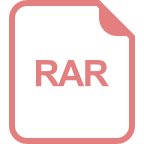
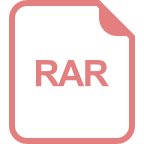
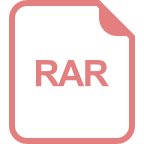
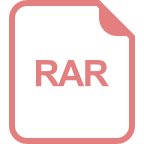
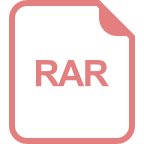
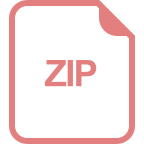
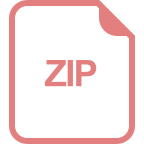
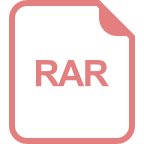
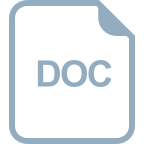
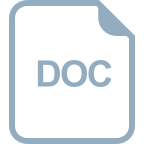
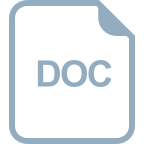
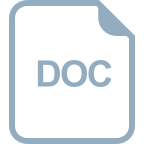