C语言如何用两个线程从一个队列取数据
时间: 2024-05-04 09:19:11 浏览: 13
C语言中可以使用线程库pthread来创建和管理线程。在使用两个线程从一个队列取数据时,可以采用生产者-消费者模型。
具体实现方法如下:
1. 定义一个队列结构体,包括队列缓冲区、队列头尾指针、队列长度等信息。
2. 创建两个线程,一个作为生产者,另一个作为消费者。
3. 生产者线程负责向队列中添加数据。生产者线程需要使用pthread_mutex_lock和pthread_mutex_unlock函数保证同步,避免多个线程同时写入数据引起冲突。
4. 消费者线程负责从队列中取出数据。消费者线程需要使用pthread_mutex_lock和pthread_mutex_unlock函数保证同步,避免多个线程同时读取数据引起冲突。
5. 通过pthread_cond_wait和pthread_cond_signal函数来实现线程之间的通信。当队列为空时,消费者线程会调用pthread_cond_wait函数进入等待状态,当队列中有数据时,生产者线程会调用pthread_cond_signal函数唤醒消费者线程。
6. 最后,记得在程序结束时释放队列和线程资源。
下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define QUEUE_SIZE 10
typedef struct {
int buffer[QUEUE_SIZE];
int head;
int tail;
int count;
pthread_mutex_t lock;
pthread_cond_t not_empty;
pthread_cond_t not_full;
} queue;
void queue_init(queue* q)
{
q->head = 0;
q->tail = 0;
q->count = 0;
pthread_mutex_init(&q->lock, NULL);
pthread_cond_init(&q->not_empty, NULL);
pthread_cond_init(&q->not_full, NULL);
}
void queue_put(queue* q, int value)
{
pthread_mutex_lock(&q->lock);
while (q->count == QUEUE_SIZE) {
pthread_cond_wait(&q->not_full, &q->lock);
}
q->buffer[q->tail] = value;
q->tail = (q->tail + 1) % QUEUE_SIZE;
q->count++;
pthread_cond_signal(&q->not_empty);
pthread_mutex_unlock(&q->lock);
}
int queue_get(queue* q)
{
pthread_mutex_lock(&q->lock);
while (q->count == 0) {
pthread_cond_wait(&q->not_empty, &q->lock);
}
int value = q->buffer[q->head];
q->head = (q->head + 1) % QUEUE_SIZE;
q->count--;
pthread_cond_signal(&q->not_full);
pthread_mutex_unlock(&q->lock);
return value;
}
void* producer(void* arg)
{
queue* q = (queue*)arg;
for (int i = 0; i < 100; i++) {
queue_put(q, i);
printf("producer: put %d\n", i);
}
return NULL;
}
void* consumer(void* arg)
{
queue* q = (queue*)arg;
for (int i = 0; i < 100; i++) {
int value = queue_get(q);
printf("consumer: get %d\n", value);
}
return NULL;
}
int main()
{
queue q;
queue_init(&q);
pthread_t tid1, tid2;
pthread_create(&tid1, NULL, producer, &q);
pthread_create(&tid2, NULL, consumer, &q);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
return 0;
}
```
在这个示例代码中,我们定义了一个队列结构体,包括队列缓冲区、队列头尾指针、队列长度等信息,并使用pthread_mutex和pthread_cond来保证线程之间的同步和通信。生产者线程负责向队列中添加数据,消费者线程负责从队列中取出数据,两个线程通过队列进行交互。
相关推荐
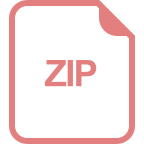
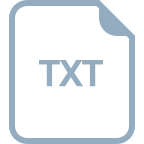
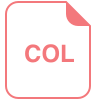
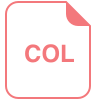
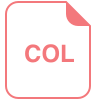
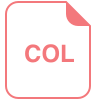
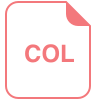









