用java写一个心形状
时间: 2023-09-12 17:05:43 浏览: 120
### 回答1:
的图案,你能给出代码吗?
可以的,以下是用 Java 编写心形图案的代码:
```
public class HeartShape {
public static void main(String[] args) {
int size = 10;
for (int y = size; y >= -size; y--) {
for (int x = -size; x <= size; x++) {
if (x * x + y * y <= size * size) {
System.out.print("* ");
} else {
System.out.print(" ");
}
}
System.out.println();
}
}
}
```
这段代码可以输出一个大小为 10 的心形图案。
### 回答2:
要用Java写一个心形状,可以使用Java中的图形处理库,例如JavaFX或者AWT。
以下是使用JavaFX库来实现的一种方法:
```java
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Path;
import javafx.stage.Stage;
public class HeartShape extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
Group root = new Group();
Scene scene = new Scene(root, 300, 300);
Path path = new Path();
path.setStroke(Color.RED);
path.setFill(Color.RED);
double centerX = scene.getWidth() / 2;
double centerY = scene.getHeight() / 2;
double radiusX = 100;
double radiusY = 100;
double controlX = centerX + 30;
double controlY = centerY - 70;
path.getElements().add(new javafx.scene.shape.MoveTo(centerX, centerY + radiusY));
path.getElements().add(new javafx.scene.shape.CubicCurveTo(centerX, centerY + radiusY,
centerX - controlX, centerY - radiusY,
centerX - radiusX, centerY));
path.getElements().add(new javafx.scene.shape.CubicCurveTo(centerX - radiusX, centerY,
centerX - radiusX, centerY - radiusY,
centerX, centerY - radiusY * 0.9));
path.getElements().add(new javafx.scene.shape.CubicCurveTo(centerX + radiusX, centerY - radiusY,
centerX + radiusX, centerY,
centerX, centerY + radiusY));
path.getElements().add(new javafx.scene.shape.CubicCurveTo(centerX, centerY + radiusY / 2,
centerX, centerY + radiusY,
centerX, centerY + radiusY));
root.getChildren().add(path);
primaryStage.setTitle("Heart Shape Example");
primaryStage.setScene(scene);
primaryStage.show();
}
}
```
这个程序使用JavaFX库创建了一个基本的Java图形界面,并在其中绘制了一个红色的心形图案。
在这个例子中,通过CubicCurveTo函数和MoveTo函数来绘制三次贝塞尔曲线来实现心形的绘制。设置不同的控制点和起点和终点来调整形状和大小。
最后,通过设置Stage和Scene来显示图形界面。
运行这个程序,你将看到一个绘制的心形图案。
### 回答3:
要用Java写一个心形状,我们可以使用绘图库来实现。在下面的代码中,我使用了Java的AWT和Swing库来画出一个心形状:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.geom.*;
public class HeartShape extends JFrame {
public HeartShape() {
super("Heart Shape");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
}
public void paint(Graphics g) {
super.paint(g);
Graphics2D g2d = (Graphics2D) g;
g2d.setColor(Color.RED);
// 使用GeneralPath构建心形图形路径
GeneralPath path = new GeneralPath();
path.moveTo(200, 250);
path.curveTo(200, 230, 150, 200, 150, 160);
path.curveTo(150, 120, 180, 90, 200, 90);
path.curveTo(220, 90, 250, 120, 250, 160);
path.curveTo(250, 200, 200, 230, 200, 250);
path.closePath();
// 填充心形图形
g2d.fill(path);
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
HeartShape hs = new HeartShape();
hs.setVisible(true);
}
});
}
}
```
这个程序创建了一个Java窗体,并在窗体上画出了一个心形状。在`paint`方法中,我们使用了Graphics2D类来绘制图形,先使用`moveTo`方法定位起始点,然后使用`curveTo`方法绘制贝塞尔曲线,最后使用`closePath`方法封闭路径。最后使用`fill`方法填充心形的内部。
运行程序后,将会显示一个红色的心形窗体。
阅读全文
相关推荐

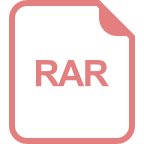



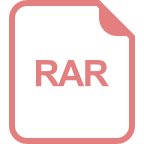
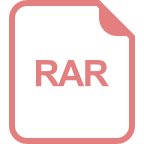

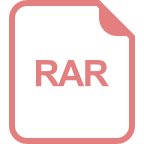
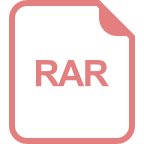
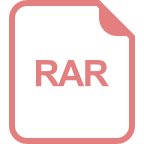
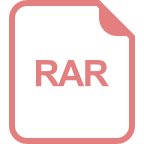


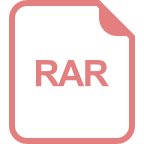
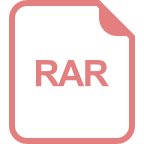