用python实现Schnorr签名完整代码
时间: 2024-10-09 13:14:17 浏览: 21
在Python中,Schnorr签名是一种基于离散对数问题的数字签名方案,它比RSA等传统签名算法更轻量级,效率更高。以下是使用Python实现Schnorr签名的一个简要示例,这里我们不会包括完整的库支持,因为这通常需要依赖于专门的安全库如`cryptography`。请注意,实际应用中,你将需要引入安全库并处理错误检查。
```python
import hashlib
from typing import Tuple
# 定义椭圆曲线参数和基本函数
def hash_to_curve(msg: bytes) -> int:
h = hashlib.sha256()
h.update(msg)
return int.from_bytes(h.digest(), 'big') % p
# 椭圆曲线点操作
def add_points(P: Tuple[int, int], Q: Tuple[int, int]) -> Tuple[int, int]:
# ... (省略复杂的椭圆曲线加法计算)
return ...
# schnorr签名
def schnorr_sign(private_key: int, msg: bytes) -> Tuple[int, int]:
r = hash_to_curve(msg)
k = private_key
R = add_points(G, k * P)
s = k + r * e
s %= n
return r, s
# 验证签名
def schnorr_verify(pub_key: Tuple[int, int], msg: bytes, signature: Tuple[int, int]) -> bool:
r, s = signature
H = hash_to_curve(msg)
u1 = s ** -1 * (H + r * pub_key[0]) % n
u2 = s ** -1 * pub_key[1] % n
V = add_points(G, u1 * P) == add_points(u2 * Q, R)
return V
# 示例
G = (p, n, P, Q) # 这些是椭圆曲线的标准参数,通常包含模p、群大小n、生成元P和基础点Q
private_key = 42 # 假设有一个私钥
msg = b'sign this message'
r, s = schnorr_sign(private_key, msg)
print(f"Signature: ({r}, {s})")
verified = schnorr_verify(G[2], msg, (r, s))
if verified:
print("Signature is valid.")
else:
print("Signature is invalid.")
阅读全文
相关推荐
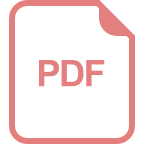
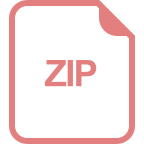















