用C语言写一个创建在D盘的文件夹
时间: 2023-05-29 12:01:53 浏览: 105
```c
#include <stdio.h>
#include <windows.h>
int main()
{
char path[] = "D:\\new_folder";
BOOL success = CreateDirectory(path, NULL);
if (success)
{
printf("Folder created successfully.");
}
else
{
printf("Failed to create folder.");
}
return 0;
}
```
注意:
1. 请在使用之前确保该路径下没有同名文件夹,否则会创建失败
2. 如果要在其他盘创建文件夹,只需要修改`path`变量的值即可例如 E盘:`char path[] = "E:\\new_folder";`
相关问题
用C语言写一个用windows.h中的头文件来实现的将D盘的data文件夹中文件按文件名称的英文不同来分类
以下是一个用C语言实现的将D盘的data文件夹中文件按文件名称的英文不同来分类的程序:
```c
#include <stdio.h>
#include <string.h>
#include <windows.h>
void classifyFiles(const char* path);
int main() {
classifyFiles("D:\\data\\");
return 0;
}
void classifyFiles(const char* path) {
char searchPath[MAX_PATH];
HANDLE hFind;
WIN32_FIND_DATAA findData;
strcpy(searchPath, path);
strcat(searchPath, "*.*");
hFind = FindFirstFileA(searchPath, &findData);
if (hFind == INVALID_HANDLE_VALUE) {
printf("Failed to find the first file!\n");
return;
}
do {
if ((findData.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY) == 0) {
char fileName[MAX_PATH];
strcpy(fileName, findData.cFileName);
int length = strlen(fileName);
if (length > 0 && fileName[length - 1] != '.') {
char folderName[2] = { fileName[0], '\0' };
char folderPath[MAX_PATH];
strcpy(folderPath, path);
strcat(folderPath, folderName);
strcat(folderPath, "\\");
CreateDirectoryA(folderPath, NULL);
char filePath[MAX_PATH];
strcpy(filePath, path);
strcat(filePath, fileName);
MoveFileA(filePath, folderPath);
}
}
} while (FindNextFileA(hFind, &findData));
FindClose(hFind);
}
```
该程序通过遍历指定目录下的所有文件,将文件按照首字母不同来分类,即将首字母为A的文件放在A文件夹下,将首字母为B的文件放在B文件夹下,以此类推。具体实现是通过创建对应的文件夹,然后将文件移动到对应的文件夹中来完成的。
linux用C语言软件实现建立一个文件夹,在此文件夹中建立一个log记录文件(也是软件实现)。 键盘随机输入一个数,通过子线程把这个数记录到log文件里面去。 同时记录输入数据的时间,并且每次输入的数据不能覆盖前面输入的数据。 编译进程需要添加参数-lpthread
以下是实现上述功能的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <pthread.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
#define MAX_SIZE 100
char folder_name[MAX_SIZE] = "my_folder"; // 文件夹名称
char log_file[MAX_SIZE]; // log文件路径
pthread_mutex_t lock; // 互斥锁,保证log文件写入的线程安全
void* log_input(void* arg) {
int num = *((int*) arg);
time_t t = time(NULL);
struct tm* tm = localtime(&t);
char time_str[MAX_SIZE];
strftime(time_str, MAX_SIZE, "%Y-%m-%d %H:%M:%S", tm); // 获取当前时间字符串
char num_str[MAX_SIZE];
sprintf(num_str, "%d", num); // 将输入的数转为字符串
pthread_mutex_lock(&lock); // 加锁
FILE* fp = fopen(log_file, "a");
if (fp == NULL) {
printf("Failed to open file: %s\n", log_file);
exit(1);
}
fprintf(fp, "%s\t%s\n", time_str, num_str); // 写入log文件
fclose(fp);
pthread_mutex_unlock(&lock); // 解锁
return NULL;
}
int main() {
// 创建文件夹
if (mkdir(folder_name, 0777) == -1) {
printf("Failed to create folder: %s\n", folder_name);
exit(1);
}
// 创建log文件
char file_name[MAX_SIZE];
time_t t = time(NULL);
struct tm* tm = localtime(&t);
strftime(file_name, MAX_SIZE, "%Y-%m-%d_%H-%M-%S.log", tm); // 获取当前时间字符串作为文件名
sprintf(log_file, "%s/%s", folder_name, file_name);
FILE* fp = fopen(log_file, "w");
if (fp == NULL) {
printf("Failed to create file: %s\n", log_file);
exit(1);
}
fclose(fp);
// 读取输入的数,并记录到log文件中
pthread_t tid;
while (1) {
int num;
scanf("%d", &num);
pthread_create(&tid, NULL, log_input, &num); // 创建子线程写入log文件
}
return 0;
}
```
在编译时需要添加参数 `-lpthread` 来链接 pthread 库。例如:
```
gcc -o my_program my_program.c -lpthread
```
程序运行后会自动创建名为 `my_folder` 的文件夹,并在其中创建以当前时间命名的log文件。每次键盘随机输入一个数后,程序会启动一个子线程将该数和当前时间记录到log文件中。由于使用了互斥锁,多个子线程同时写入log文件时不会发生竞争条件。
阅读全文
相关推荐


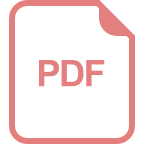
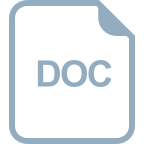
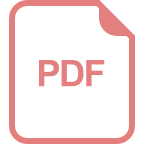
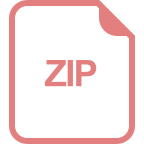
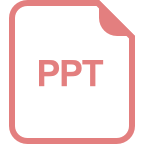
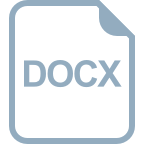
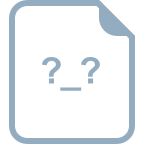
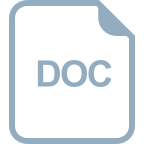
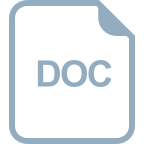
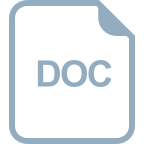
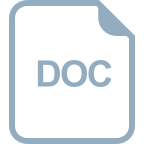
