用java语言使用Cipher实现RSA算法并对文件加密
时间: 2024-02-25 16:55:40 浏览: 141
好的,我可以为您提供一些参考代码。首先,我们需要生成RSA公钥和私钥,可以使用Java提供的KeyPairGenerator类来实现。以下是一个示例代码:
```java
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.NoSuchAlgorithmException;
public class RSAKeyGenerator {
public static KeyPair generateKeyPair() throws NoSuchAlgorithmException {
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048);
return keyPairGenerator.generateKeyPair();
}
}
```
在上面的代码中,我们使用2048位的RSA算法生成公钥和私钥。接下来,我们可以使用Cipher类来实现加密和解密。以下是一个示例代码:
```java
import javax.crypto.Cipher;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.security.Key;
import java.security.KeyPair;
import java.security.PrivateKey;
import java.security.PublicKey;
public class RSAFileEncryptor {
public static void encrypt(String inputFilePath, String outputFilePath, PublicKey publicKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
Path inputPath = Paths.get(inputFilePath);
byte[] inputBytes = Files.readAllBytes(inputPath);
byte[] outputBytes = cipher.doFinal(inputBytes);
Path outputPath = Paths.get(outputFilePath);
Files.write(outputPath, outputBytes);
}
public static void decrypt(String inputFilePath, String outputFilePath, PrivateKey privateKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
Path inputPath = Paths.get(inputFilePath);
byte[] inputBytes = Files.readAllBytes(inputPath);
byte[] outputBytes = cipher.doFinal(inputBytes);
Path outputPath = Paths.get(outputFilePath);
Files.write(outputPath, outputBytes);
}
}
```
在上面的代码中,我们使用Cipher类的init方法来初始化加密和解密模式,并使用doFinal方法来执行加密和解密操作。我们可以将输入文件的内容读入字节数组中,使用Cipher类对其加密或解密,然后将结果写入输出文件中。
使用示例:
```java
import java.security.KeyPair;
public class Main {
public static void main(String[] args) throws Exception {
KeyPair keyPair = RSAKeyGenerator.generateKeyPair();
RSAFileEncryptor.encrypt("input.txt", "output.txt", keyPair.getPublic());
RSAFileEncryptor.decrypt("output.txt", "decrypted.txt", keyPair.getPrivate());
}
}
```
在上面的示例中,我们生成了一个RSA密钥对,并使用公钥加密了名为“input.txt”的文件,并将结果写入名为“output.txt”的文件中。然后,我们使用私钥对“output.txt”文件进行解密,并将结果写入名为“decrypted.txt”的文件中。
阅读全文
相关推荐
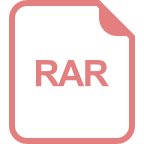
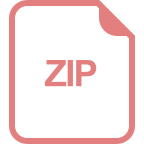
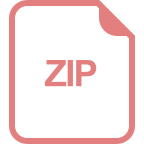
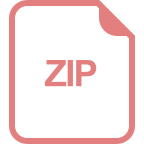
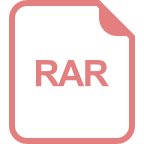
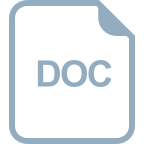
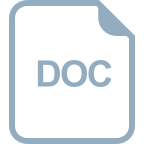
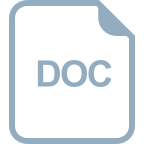






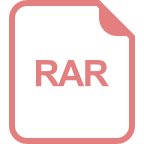
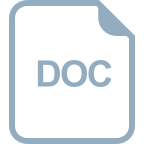