用Java实现 rsa加密算法
时间: 2023-10-30 11:07:51 浏览: 91
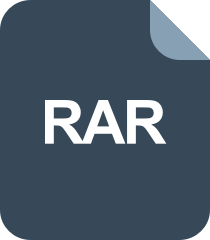
java实现RSA加密算法

RSA加密算法是一种非常常见的加密算法,Java中也提供了相关的API实现。以下是用Java实现RSA加密算法的示例代码:
```java
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.SecureRandom;
import javax.crypto.Cipher;
public class RSAEncryptionExample {
private static final String ALGORITHM = "RSA";
public static void main(String[] args) throws Exception {
// 生成RSA密钥对
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance(ALGORITHM);
SecureRandom secureRandom = new SecureRandom();
keyPairGenerator.initialize(2048, secureRandom);
KeyPair keyPair = keyPairGenerator.generateKeyPair();
PrivateKey privateKey = keyPair.getPrivate();
PublicKey publicKey = keyPair.getPublic();
// 加密数据
String plainText = "Hello, world!";
byte[] cipherText = encrypt(plainText.getBytes(), publicKey);
// 解密数据
byte[] decryptedText = decrypt(cipherText, privateKey);
System.out.println(new String(decryptedText));
}
public static byte[] encrypt(byte[] data, PublicKey publicKey) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(data);
}
public static byte[] decrypt(byte[] data, PrivateKey privateKey) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, privateKey);
return cipher.doFinal(data);
}
}
```
在本示例中,我们首先使用`KeyPairGenerator`生成一个RSA密钥对,然后使用`encrypt`和`decrypt`方法进行加密和解密操作。需要注意的是,在实际使用中,我们通常不会直接使用RSA加密大数据,而是使用RSA加密AES密钥等方式进行加密保护。
阅读全文
相关推荐


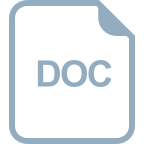
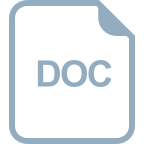
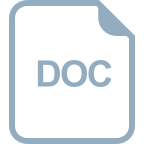
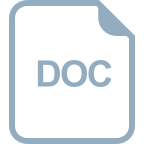
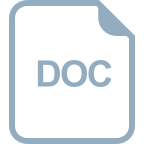
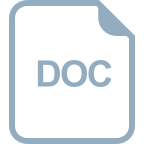
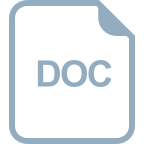
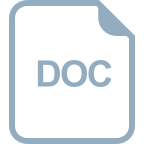

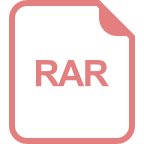
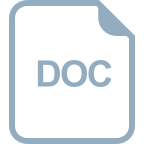
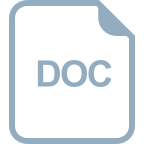
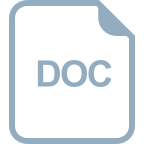