用Java实现 rsa加密算法 用中文注释
时间: 2024-05-05 08:21:19 浏览: 8
以下是Java实现RSA加密算法的示例代码,注释中有中文解释:
```java
import java.math.BigInteger;
import java.security.SecureRandom;
public class RSA {
private final static BigInteger one = new BigInteger("1");
private final static SecureRandom random = new SecureRandom();
private BigInteger privateKey;
private BigInteger publicKey;
private BigInteger modulus;
// 生成公钥和私钥
public RSA(int bitLength) {
BigInteger p = BigInteger.probablePrime(bitLength / 2, random);
BigInteger q = BigInteger.probablePrime(bitLength / 2, random);
BigInteger phi = (p.subtract(one)).multiply(q.subtract(one));
modulus = p.multiply(q);
publicKey = new BigInteger("65537"); // 一般采用65537作为公钥
privateKey = publicKey.modInverse(phi);
}
// 加密
public byte[] encrypt(byte[] message) {
return (new BigInteger(message)).modPow(publicKey, modulus).toByteArray();
}
// 解密
public byte[] decrypt(byte[] message) {
return (new BigInteger(message)).modPow(privateKey, modulus).toByteArray();
}
}
```
使用示例:
```java
public class Main {
public static void main(String[] args) {
RSA rsa = new RSA(1024);
String message = "Hello RSA!";
System.out.println("原文:" + message);
byte[] encrypted = rsa.encrypt(message.getBytes());
System.out.println("加密后:" + new String(encrypted));
byte[] decrypted = rsa.decrypt(encrypted);
System.out.println("解密后:" + new String(decrypted));
}
}
```
相关推荐
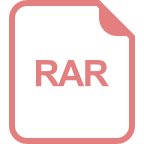
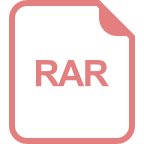
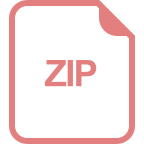














