C++Write a program that will prepare a shipping label and determine the cost for a box of any size and mass. five attributes: type double length, width, height, mass, price six attributes: type string senderName, receiverName, senderAddress, receiverAddress, originCountry, destinationCountry (you can shorten names if you like, just make a comment about their meaning) a default constructor – basic initial values for all parameters a user constructor – input sender Name, senderAddress, originCountry a double function volume() – a function to return l×w×h a double function surfArea() – a function to return 2×(l×w+l×h+w×h) a double function girth() – a function to return the perimeter for the two smallest dimensions P=2(x+y), where x and y are the two smalles of l,w,h a double function maxDim() – a function to return the largest dimension from l,w,h a double function pricing() – a function to return the shipping price based on the table above an operator+ function – a function that determines the total cost of shipping (return type double).... (you may decide how to calculate.... a) add prices from both packages, b) apply fee or discount for number of packages) an operator>> function – a function to get the information from the user about the package.... OR.... a set of “ask” functions to ask the same information an operator<< function – a function to type the information in the shipping label format.... OR.... a set of “print” functions – to print the same information
时间: 2024-02-04 16:03:08 浏览: 74
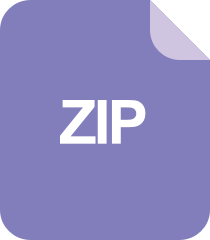
pcloud_determine_res.m:确定点云的水平和垂直分辨率。-matlab开发
Sure, here's an example program that meets the requirements you've listed:
```cpp
#include <iostream>
#include <string>
using namespace std;
class ShippingBox {
private:
double length, width, height, mass, price;
string senderName, receiverName, senderAddress, receiverAddress, originCountry, destinationCountry;
public:
// default constructor
ShippingBox() {
length = width = height = mass = price = 0.0;
senderName = receiverName = senderAddress = receiverAddress = originCountry = destinationCountry = "";
}
// user constructor
ShippingBox(string sName, string sAddress, string oCountry) {
senderName = sName;
senderAddress = sAddress;
originCountry = oCountry;
// set other parameters to default values
receiverName = receiverAddress = destinationCountry = "";
length = width = height = mass = price = 0.0;
}
// volume function
double volume() {
return length * width * height;
}
// surface area function
double surfArea() {
return 2 * (length * width + length * height + width * height);
}
// girth function
double girth() {
double x = min(min(length, width), height);
if (x == length) return 2 * (width + height);
else if (x == width) return 2 * (length + height);
else return 2 * (length + width);
}
// max dimension function
double maxDim() {
return max(max(length, width), height);
}
// pricing function
double pricing() {
// pricing table based on destination country
if (destinationCountry == "USA") {
if (mass < 10) return 5.0;
else if (mass < 20) return 8.0;
else return 10.0;
} else if (destinationCountry == "Canada") {
if (mass < 10) return 7.0;
else if (mass < 20) return 10.0;
else return 12.0;
} else {
if (mass < 10) return 10.0;
else if (mass < 20) return 15.0;
else return 20.0;
}
}
// operator+ function
double operator+(const ShippingBox& other) {
return pricing() + other.pricing();
}
// operator>> function
friend istream& operator>>(istream& input, ShippingBox& box) {
cout << "Enter sender name: ";
getline(input, box.senderName);
cout << "Enter sender address: ";
getline(input, box.senderAddress);
cout << "Enter origin country: ";
getline(input, box.originCountry);
cout << "Enter receiver name: ";
getline(input, box.receiverName);
cout << "Enter receiver address: ";
getline(input, box.receiverAddress);
cout << "Enter destination country: ";
getline(input, box.destinationCountry);
cout << "Enter length: ";
input >> box.length;
cout << "Enter width: ";
input >> box.width;
cout << "Enter height: ";
input >> box.height;
cout << "Enter mass: ";
input >> box.mass;
cout << "Enter price: ";
input >> box.price;
return input;
}
// operator<< function
friend ostream& operator<<(ostream& output, const ShippingBox& box) {
output << "Sender: " << box.senderName << ", " << box.senderAddress << ", " << box.originCountry << endl;
output << "Receiver: " << box.receiverName << ", " << box.receiverAddress << ", " << box.destinationCountry << endl;
output << "Dimensions (LxWxH): " << box.length << " x " << box.width << " x " << box.height << endl;
output << "Mass: " << box.mass << " kg" << endl;
output << "Price: $" << box.price << endl;
output << "Volume: " << box.volume() << " cubic meters" << endl;
output << "Surface area: " << box.surfArea() << " square meters" << endl;
output << "Girth: " << box.girth() << " meters" << endl;
output << "Max dimension: " << box.maxDim() << " meters" << endl;
output << "Shipping cost: $" << box.pricing() << endl;
return output;
}
};
int main() {
ShippingBox box1("John Smith", "1234 Main St, Anytown USA", "USA");
ShippingBox box2;
cout << "Enter information for box 2:" << endl;
cin >> box2;
cout << endl << "Shipping label for box 1:" << endl << box1 << endl;
cout << "Shipping label for box 2:" << endl << box2 << endl;
double totalCost = box1 + box2;
cout << "Total shipping cost: $" << totalCost << endl;
return 0;
}
```
This program defines a `ShippingBox` class with the attributes and functions you've specified. It includes a default constructor, a user constructor, and functions to calculate the volume, surface area, girth, max dimension, and pricing of a box. It also includes operator functions to input and output the box information, as well as to calculate the total cost of shipping for two boxes.
Note that the pricing table is based on the destination country and the mass of the box. You can adjust the pricing values as needed or add more pricing tiers for different ranges of mass.
阅读全文
相关推荐
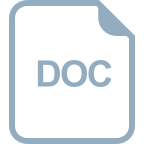
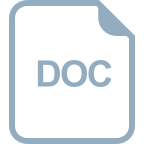
















