基于DES算法的OFB工作模式演示程序实现
时间: 2023-10-23 12:09:45 浏览: 124
这里提供一个基于Python语言实现的基于DES算法的OFB工作模式演示程序。请注意,该程序仅供学习和研究使用,不可用于商业和其他非法用途。
```python
from Crypto.Cipher import DES
from Crypto.Util.Padding import pad, unpad
import tkinter as tk
class OFB_GUI:
def __init__(self):
self.root = tk.Tk()
self.root.title("OFB模式演示程序")
self.root.geometry("600x400")
# 加密区域
self.encrypt_frame = tk.Frame(self.root, width=250, height=300)
self.encrypt_frame.place(x=20, y=20)
tk.Label(self.encrypt_frame, text="加密", font=("Arial", 16)).place(x=100, y=10)
tk.Label(self.encrypt_frame, text="明文", font=("Arial", 12)).place(x=10, y=60)
tk.Label(self.encrypt_frame, text="密钥", font=("Arial", 12)).place(x=10, y=100)
tk.Label(self.encrypt_frame, text="IV", font=("Arial", 12)).place(x=10, y=140)
self.encrypt_text = tk.Text(self.encrypt_frame, width=20, height=5)
self.encrypt_text.place(x=80, y=60)
self.encrypt_key = tk.Entry(self.encrypt_frame, width=20)
self.encrypt_key.place(x=80, y=100)
self.encrypt_iv = tk.Entry(self.encrypt_frame, width=20)
self.encrypt_iv.place(x=80, y=140)
self.encrypt_button = tk.Button(self.encrypt_frame, text="加密", command=self.encrypt)
self.encrypt_button.place(x=100, y=200)
tk.Label(self.encrypt_frame, text="密文", font=("Arial", 12)).place(x=10, y=260)
self.encrypt_result = tk.Text(self.encrypt_frame, width=20, height=5)
self.encrypt_result.place(x=80, y=260)
# 解密区域
self.decrypt_frame = tk.Frame(self.root, width=250, height=300)
self.decrypt_frame.place(x=330, y=20)
tk.Label(self.decrypt_frame, text="解密", font=("Arial", 16)).place(x=100, y=10)
tk.Label(self.decrypt_frame, text="密文", font=("Arial", 12)).place(x=10, y=60)
tk.Label(self.decrypt_frame, text="密钥", font=("Arial", 12)).place(x=10, y=100)
tk.Label(self.decrypt_frame, text="IV", font=("Arial", 12)).place(x=10, y=140)
self.decrypt_text = tk.Text(self.decrypt_frame, width=20, height=5)
self.decrypt_text.place(x=80, y=60)
self.decrypt_key = tk.Entry(self.decrypt_frame, width=20)
self.decrypt_key.place(x=80, y=100)
self.decrypt_iv = tk.Entry(self.decrypt_frame, width=20)
self.decrypt_iv.place(x=80, y=140)
self.decrypt_button = tk.Button(self.decrypt_frame, text="解密", command=self.decrypt)
self.decrypt_button.place(x=100, y=200)
tk.Label(self.decrypt_frame, text="明文", font=("Arial", 12)).place(x=10, y=260)
self.decrypt_result = tk.Text(self.decrypt_frame, width=20, height=5)
self.decrypt_result.place(x=80, y=260)
self.root.mainloop()
def encrypt(self):
plaintext = self.encrypt_text.get('1.0', 'end').encode('utf-8')
key = self.encrypt_key.get().encode('utf-8')
iv = self.encrypt_iv.get().encode('utf-8')
cipher = DES.new(key, DES.MODE_OFB, iv)
ciphertext = cipher.encrypt(pad(plaintext, DES.block_size))
self.encrypt_result.delete('1.0', 'end')
self.encrypt_result.insert('end', ciphertext.hex())
def decrypt(self):
ciphertext = bytes.fromhex(self.decrypt_text.get('1.0', 'end').strip())
key = self.decrypt_key.get().encode('utf-8')
iv = self.decrypt_iv.get().encode('utf-8')
cipher = DES.new(key, DES.MODE_OFB, iv)
plaintext = unpad(cipher.decrypt(ciphertext), DES.block_size)
self.decrypt_result.delete('1.0', 'end')
self.decrypt_result.insert('end', plaintext.decode('utf-8'))
if __name__ == '__main__':
ofb_gui = OFB_GUI()
```
在该程序中,使用了Crypto库中的DES算法和Padding模块,以及Tkinter库实现了图形化界面。可以通过输入明文、密钥和IV来进行加密和解密。
阅读全文
相关推荐
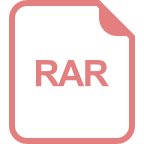
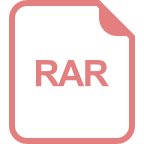

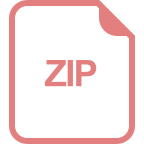
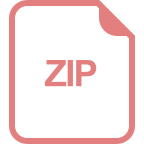
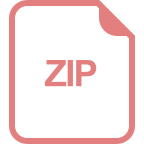
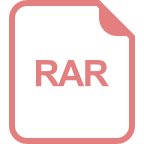
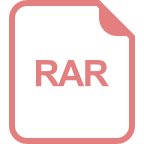
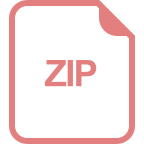
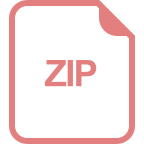
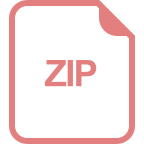
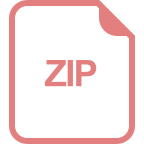
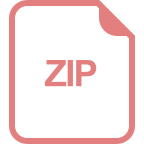
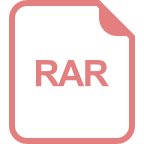
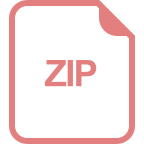
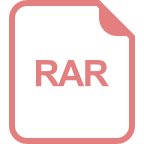
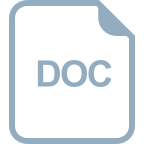
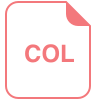