java如何实现 超大文本正则快速匹配
时间: 2023-07-07 07:31:29 浏览: 53
超大文本的正则快速匹配需要综合考虑以下几个因素:
1. 分段处理:超大文本无法一次性读入内存,因此需要分段读取并处理。可以使用BufferedReader按行读取文本,并对每一行文本进行正则匹配。
2. 正则表达式优化:正则表达式的效率对于超大文本的处理尤为重要,需要进行正则表达式的优化。可以使用String类的方法进行简单的匹配,例如contains()、startsWith()、endsWith()等方法。
3. 多线程处理:如果文本较大,可以考虑使用多线程进行处理,提高匹配效率。可以将文本分成多个块,每个线程处理一个块。
下面是一个示例代码,演示如何实现超大文本正则快速匹配:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class LargeTextRegexMatch {
public static void main(String[] args) throws IOException {
String filePath = "large_text_file.txt";
String pattern = "test";
Pattern p = Pattern.compile(pattern);
// 使用BufferedReader进行缓存读取
try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) {
String line;
List<String> lines = new ArrayList<>();
while ((line = reader.readLine()) != null) {
lines.add(line);
}
// 使用多线程进行处理
int threads = Runtime.getRuntime().availableProcessors();
ExecutorService executor = Executors.newFixedThreadPool(threads);
int blockSize = lines.size() / threads;
for (int i = 0; i < threads; i++) {
int start = i * blockSize;
int end = i == threads - 1 ? lines.size() : (i + 1) * blockSize;
executor.execute(() -> {
Matcher m = p.matcher("");
for (int j = start; j < end; j++) {
String lineToMatch = lines.get(j);
// 将每一行文本添加到Matcher对象中
m.reset(lineToMatch);
while (m.find()) {
System.out.println("Matched: " + m.group());
}
}
});
}
executor.shutdown();
}
}
}
```
在这个示例代码中,我们首先将文本按行读取,并保存到一个List中。然后,使用多线程进行处理,将文本分成多个块,每个线程处理一个块。在每个线程中,使用Matcher对象对每一行文本进行正则匹配,匹配过程与前面的示例代码相同。通过多线程处理和分块处理,可以提高处理超大文本的效率。
相关推荐
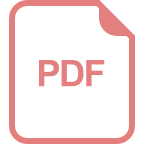
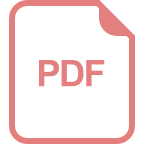














