请用threejs写一个网页完整代码,立方体绕指定两点所在直线旋转
时间: 2023-06-13 18:06:58 浏览: 60
以下是一个使用Three.js库实现立方体绕指定两点所在直线旋转的完整网页代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Three.js Cube Rotation</title>
<style>
body {
margin: 0;
overflow: hidden;
}
canvas {
display: block;
}
</style>
</head>
<body>
<script src="https://cdn.jsdelivr.net/npm/three@0.128.0/build/three.min.js"></script>
<script>
// Create scene, camera and renderer
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// Create cube and add to scene
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
// Set up variables for rotation animation
const axis = new THREE.Vector3();
const pointA = new THREE.Vector3(0, 0, 0);
const pointB = new THREE.Vector3(5, 5, 5);
const angle = Math.PI / 2;
let t = 0;
// Function to rotate cube around line between pointA and pointB
function rotateAroundLine() {
// Calculate axis of rotation
axis.subVectors(pointB, pointA).normalize();
// Set quaternion for current rotation
const quaternion = new THREE.Quaternion().setFromAxisAngle(axis, angle * t);
// Apply quaternion to cube's rotation
cube.quaternion.copy(quaternion);
// Increment t for next frame
t += 0.01;
}
// Render loop
function animate() {
requestAnimationFrame(animate);
rotateAroundLine();
renderer.render(scene, camera);
}
animate();
// Resize handler
window.addEventListener('resize', () => {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
});
</script>
</body>
</html>
```
在这个示例中,我们创建了一个Three.js场景并添加了一个绿色立方体。我们使用了一个`rotateAroundLine`函数来计算立方体当前帧应该绕直线旋转多少,并且在每一帧中都将其应用到立方体的旋转上。我们还在`animate`函数中调用`rotateAroundLine`函数,并使用`requestAnimationFrame`来启动渲染循环。最后,我们添加了一个`resize`事件处理程序,以确保在窗口大小改变时,相机和渲染器的视口与新的窗口大小匹配。
相关推荐
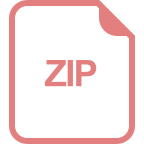
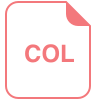













