五子棋人机对弈python
时间: 2023-10-08 13:09:03 浏览: 122
实现五子棋人机对弈的方法有很多,以下是一种基于Python的实现方法:
1. 安装Pygame库,用于图形界面的显示和交互。
```
pip install pygame
```
2. 创建一个棋盘类,用于绘制棋盘和管理棋子的落子情况。
```
class Chessboard:
def __init__(self):
self.width = 600
self.height = 600
self.grid_size = 40
self.rows = self.width // self.grid_size
self.cols = self.height // self.grid_size
self.grid = [[0 for x in range(self.cols)] for y in range(self.rows)]
self.player = 1
self.game_over = False
self.winner = None
self.font = pygame.font.SysFont("Arial", 48)
```
3. 创建一个游戏类,用于处理游戏的主逻辑和事件响应。
```
class Game:
def __init__(self):
self.screen = pygame.display.set_mode((600, 600))
pygame.display.set_caption("五子棋")
self.clock = pygame.time.Clock()
self.chessboard = Chessboard()
def run(self):
while not self.chessboard.game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
if self.chessboard.player == 1:
self.chessboard.drop_chess(x, y)
self.chessboard.player = 2
if self.chessboard.check_win():
self.chessboard.game_over = True
self.chessboard.winner = 1
elif self.chessboard.player == 2:
# AI下棋
x, y = self.ai_move()
self.chessboard.drop_chess(x, y)
self.chessboard.player = 1
if self.chessboard.check_win():
self.chessboard.game_over = True
self.chessboard.winner = 2
self.screen.fill((255, 255, 255))
self.chessboard.draw(self.screen)
pygame.display.update()
self.clock.tick(60)
self.show_result()
def ai_move(self):
# TODO: 实现AI下棋的算法
pass
def show_result(self):
if self.chessboard.winner == 1:
text = self.chessboard.font.render("玩家1获胜", True, (255, 0, 0))
elif self.chessboard.winner == 2:
text = self.chessboard.font.render("玩家2获胜", True, (255, 0, 0))
else:
text = self.chessboard.font.render("平局", True, (255, 0, 0))
self.screen.blit(text, (150, 250))
pygame.display.update()
pygame.time.delay(3000)
```
4. 实现AI下棋的算法。可以使用基于博弈树的搜索算法,或者基于神经网络的强化学习算法等。
```
def ai_move(self):
# TODO: 实现AI下棋的算法
# 这里暂时使用随机下棋的方式
while True:
x = random.randint(0, self.chessboard.cols - 1)
y = random.randint(0, self.chessboard.rows - 1)
if self.chessboard.grid[y][x] == 0:
return x, y
```
5. 在主函数中,创建游戏对象并启动游戏。
```
if __name__ == '__main__':
pygame.init()
game = Game()
game.run()
```
以上是一个简单的五子棋人机对弈的实现方法,可以根据需要进行扩展和优化。
阅读全文
相关推荐
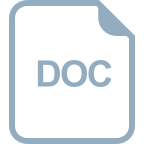
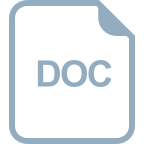
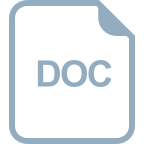
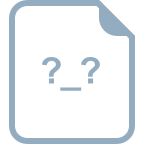



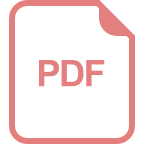
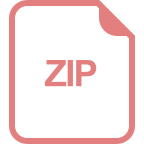
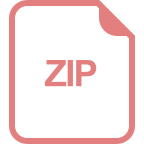
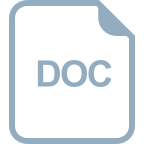
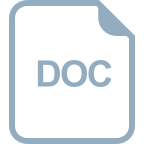





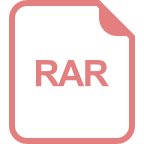