优先级调度算法用java实现
时间: 2023-09-30 09:08:40 浏览: 76
优先级调度算法是一种常用的进程调度算法,它根据进程的优先级来决定下一个要执行的进程。以下是用Java实现优先级调度算法的基本步骤:
1. 定义进程类:包括进程ID、进程优先级、进程执行时间等属性。
2. 构建进程队列:将所有进程按照优先级从高到低排序,并加入到进程队列中。
3. 执行进程:从进程队列中选取优先级最高的进程执行,执行完毕后将进程从队列中移除。
4. 更新进程优先级:如果一个进程执行完毕后,其优先级没有发生变化,则将其优先级降低一个级别;如果一个进程因等待I/O而阻塞,则将其优先级提高一个级别。
以下是一个简单的Java代码示例,用于实现优先级调度算法:
```java
public class PriorityScheduling {
//定义进程类
static class Process {
private int id; //进程ID
private int priority; //进程优先级
private int burstTime; //进程执行时间
public Process(int id, int priority, int burstTime) {
this.id = id;
this.priority = priority;
this.burstTime = burstTime;
}
public int getId() {
return id;
}
public int getPriority() {
return priority;
}
public int getBurstTime() {
return burstTime;
}
public void setPriority(int priority) {
this.priority = priority;
}
public void setBurstTime(int burstTime) {
this.burstTime = burstTime;
}
}
//构建进程队列
public static List<Process> buildProcessQueue() {
List<Process> processQueue = new ArrayList<>();
//初始化进程队列
return processQueue;
}
//执行进程
public static void executeProcess(List<Process> processQueue) {
int currentTime = 0;
while (!processQueue.isEmpty()) {
Process process = getHighestPriorityProcess(processQueue);
processQueue.remove(process);
System.out.println("Time " + currentTime + ": Process " + process.getId() + " is executed.");
currentTime += process.getBurstTime();
updateProcessPriority(processQueue, process);
}
}
//获取优先级最高的进程
public static Process getHighestPriorityProcess(List<Process> processQueue) {
Process highestPriorityProcess = null;
for (Process process : processQueue) {
if (highestPriorityProcess == null || process.getPriority() > highestPriorityProcess.getPriority()) {
highestPriorityProcess = process;
}
}
return highestPriorityProcess;
}
//更新进程优先级
public static void updateProcessPriority(List<Process> processQueue, Process process) {
for (Process p : processQueue) {
if (p == process) {
continue;
}
if (p.getPriority() > 1) {
p.setPriority(p.getPriority() - 1);
}
}
}
//主函数
public static void main(String[] args) {
List<Process> processQueue = buildProcessQueue();
executeProcess(processQueue);
}
}
```
需要注意的是,以上代码只是一个简单的示例,实际应用中需要根据具体问题进行修改和优化。
相关推荐
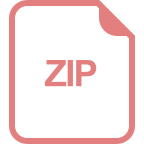
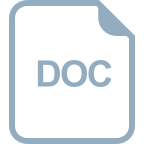














