c语言代码用顺序表求两集合交集
时间: 2024-05-13 16:15:40 浏览: 195
假设两个集合A和B已经存储在顺序表中,可以按照以下步骤求它们的交集:
1.定义一个新的顺序表C,用于存储交集结果;
2.遍历集合A,对于A中的每个元素,遍历集合B,查找是否存在相同的元素,若存在则将该元素插入到C中;
3.遍历完成后,C中存储的即为A和B的交集。
以下是求两集合交集的C语言代码实现:
```c
#include <stdio.h>
#define MAXSIZE 100
// 定义顺序表
typedef struct {
int data[MAXSIZE];
int length;
} SqList;
// 初始化顺序表
void InitList(SqList *L) {
L->length = 0;
}
// 插入元素
void InsertElem(SqList *L, int elem) {
if (L->length >= MAXSIZE) {
printf("List is full!\n");
return;
}
L->data[L->length] = elem;
L->length++;
}
// 求两集合交集
void GetIntersection(SqList *A, SqList *B, SqList *C) {
InitList(C);
int i, j;
for (i = 0; i < A->length; i++) {
for (j = 0; j < B->length; j++) {
if (A->data[i] == B->data[j]) {
InsertElem(C, A->data[i]);
break;
}
}
}
}
// 输出顺序表元素
void PrintList(SqList *L) {
int i;
for (i = 0; i < L->length; i++) {
printf("%d ", L->data[i]);
}
printf("\n");
}
int main() {
SqList A = {{1, 3, 5, 7, 9}, 5};
SqList B = {{2, 3, 5, 7, 8}, 5};
SqList C;
GetIntersection(&A, &B, &C);
printf("A ∩ B: ");
PrintList(&C);
return 0;
}
```
输出结果为:
```
A ∩ B: 3 5 7
```
阅读全文
相关推荐







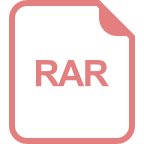










